本文列舉了Direct3D中各種紋理應用實現:黑暗貼圖,發光貼圖,漫反射映射貼圖,細節紋理,紋理混合,有較詳盡的注解。其中黑暗貼圖,發光貼圖,細節紋理都是采用多重紋理的方法實現(也可以采用多次渲染混合實現)。
示例代碼使用Beginning direct3D game programming中的框架,省去不少事情,可以專注紋理話題。代碼:
點此下載下面來看代碼與效果:
正常的紋理貼圖效果:
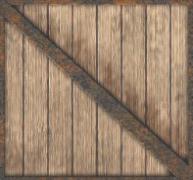
正常的紋理貼圖代碼:
1
//基本紋理
2
void drawNormalTexture()
3

{
4
// 設置box紋理貼圖
5
Device->SetTexture(0, texBox);
6
Device->SetTextureStageState(0, D3DTSS_TEXCOORDINDEX, 0); //使用紋理坐標
7
Device->SetTextureStageState(0, D3DTSS_COLORARG1, D3DTA_TEXTURE); // 顏色來源-材質
8
Device->SetTextureStageState(0, D3DTSS_COLOROP, D3DTOP_SELECTARG1); // 使用當前顏色作為第一個texture stage的輸出
9
10
// 描繪box
11
Box->draw(0, 0, 0);
12
}
黑暗紋理貼圖效果:
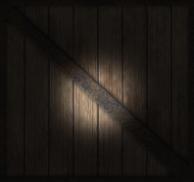
黑暗紋理貼圖代碼:
1
//黑暗映射紋理
2
void drawDarkMapTexture()
3

{
4
// Multi texture:多重紋理,此處為兩重紋理
5
// finalColor = destPixelColor * sourcePixelColor
6
// 設置box紋理貼圖
7
Device->SetTexture(0, texBox);
8
Device->SetTextureStageState(0, D3DTSS_TEXCOORDINDEX, 0);
9
Device->SetTextureStageState(0, D3DTSS_COLORARG1, D3DTA_TEXTURE); // 顏色來源-材質
10
Device->SetTextureStageState(0, D3DTSS_COLOROP, D3DTOP_SELECTARG1); // 使用當前顏色作為第一個texture stage的輸出
11
12
// 設置黑暗紋理貼圖
13
Device->SetTexture(1, texAlpha);
14
Device->SetTextureStageState(1, D3DTSS_TEXCOORDINDEX, 0);
15
Device->SetTextureStageState(1, D3DTSS_COLORARG1, D3DTA_TEXTURE); // 顏色來源-材質
16
Device->SetTextureStageState(1, D3DTSS_COLORARG2, D3DTA_CURRENT); // 顏色來源-前一個texture stage
17
Device->SetTextureStageState(1, D3DTSS_COLOROP, D3DTOP_MODULATE); // 顏色混合:相乘
18
19
// 描繪box
20
Box->draw(0, 0, 0);
21
}
漫反射映射貼圖效果:夜光鏡效果
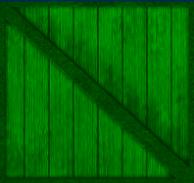
漫反射映射貼圖代碼:
1
//漫射光映射紋理
2
void drawDiffuseTexture()
3

{
4
// 設置box紋理貼圖
5
Device->SetTexture(0, texBox);
6
Device->SetTextureStageState(0, D3DTSS_TEXCOORDINDEX, 0);
7
Device->SetTextureStageState(0, D3DTSS_COLORARG1, D3DTA_TEXTURE); // 顏色來源-材質
8
Device->SetTextureStageState(0, D3DTSS_COLORARG2, D3DTA_DIFFUSE); // 顏色來源-漫反射
9
Device->SetTextureStageState(0, D3DTSS_COLOROP, D3DTOP_MODULATE); // 顏色混合
10
11
// 設置材質:綠色材質實現類似夜光鏡的效果
12
Device->SetMaterial(&d3d::GREEN_MTRL);
13
14
// 描繪box
15
Box->draw(0, 0, 0);
16
}
發光映射紋理貼圖效果:
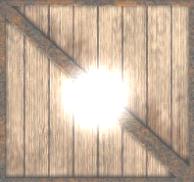
發光映射紋理貼圖代碼:
1
//發光映射紋理
2
void drawGlowMapTexture()
3

{
4
// Multi texture:多重紋理,此處為兩重紋理
5
// finalColor = sourcePixelColor * 1.0 + destPixelColor * 1.0
6
// 設置box紋理貼圖
7
Device->SetTexture(0, texBox);
8
Device->SetTextureStageState(0, D3DTSS_TEXCOORDINDEX, 0);
9
Device->SetTextureStageState(0, D3DTSS_COLORARG1, D3DTA_TEXTURE); // 顏色來源-材質
10
Device->SetTextureStageState(0, D3DTSS_COLOROP, D3DTOP_SELECTARG1); // 使用當前顏色作為第一個texture stage的輸出
11
12
// 設置黑暗紋理貼圖
13
Device->SetTexture(1, texAlpha);
14
Device->SetTextureStageState(1, D3DTSS_TEXCOORDINDEX, 0);
15
Device->SetTextureStageState(1, D3DTSS_COLORARG1, D3DTA_TEXTURE); // 顏色來源-材質
16
Device->SetTextureStageState(1, D3DTSS_COLORARG2, D3DTA_CURRENT); // 顏色來源-前一個texture stage
17
Device->SetTextureStageState(1, D3DTSS_COLOROP, D3DTOP_ADD); // 顏色混合:相加
18
19
// 描繪box
20
Box->draw(0, 0, 0);
21
}
細節映射紋理貼圖:實現粗糙的凹凸效果
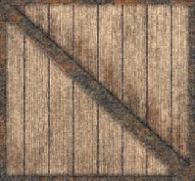
細節映射紋理貼圖代碼:
1
//細節映射紋理:實現凹凸效果
2
void drawDetailMapTexture()
3

{
4
// Multi texture:多重紋理,此處為兩重紋理
5
// finalColor = sourcePixelColor * destPixelColor + destPixelColor * sourcePixelColor
6
// 設置box紋理貼圖
7
Device->SetTexture(0, texBox);
8
Device->SetTextureStageState(0, D3DTSS_TEXCOORDINDEX, 0);
9
Device->SetTextureStageState(0, D3DTSS_COLORARG1, D3DTA_TEXTURE); // 顏色來源-材質
10
Device->SetTextureStageState(0, D3DTSS_COLOROP, D3DTOP_SELECTARG1); // 使用當前顏色作為第一個texture stage的輸出
11
12
// 設置細節紋理貼圖
13
Device->SetTexture(1, texDetail);
14
Device->SetTextureStageState(1, D3DTSS_TEXCOORDINDEX, 0);
15
Device->SetTextureStageState(1, D3DTSS_COLORARG1, D3DTA_TEXTURE); // 顏色來源-材質
16
Device->SetTextureStageState(1, D3DTSS_COLORARG2, D3DTA_CURRENT); // 顏色來源-前一個渲染通道
17
Device->SetTextureStageState(1, D3DTSS_COLOROP, D3DTOP_ADDSIGNED); // 顏色混合
18
19
// 描繪box
20
Box->draw(0, 0, 0);
21
}
alpha紋理混合效果:多次渲染實現
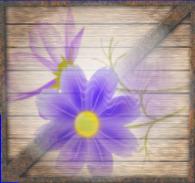
alph紋理混合代碼:
1
//alpha混合紋理
2
void drawAlphaBlendTexture()
3

{
4
// 多次渲染實現紋理混合
5
// finalColor = sourcePixelColor * sourceBlendFactor + destPixelColor * destBlendFactor
6
// 設置紋理混合參數
7
Device->SetTextureStageState(0, D3DTSS_ALPHAARG1, D3DTA_TEXTURE); // alpha值來自紋理
8
Device->SetTextureStageState(0, D3DTSS_ALPHAOP, D3DTOP_SELECTARG1);
9
10
// 設置混合因子實現透明效果
11
Device->SetRenderState(D3DRS_SRCBLEND, D3DBLEND_SRCALPHA);
12
Device->SetRenderState(D3DRS_DESTBLEND, D3DBLEND_INVSRCALPHA);
13
14
//使用box紋理貼圖實現第一次渲染,無alpha混合
15
Device->SetTexture(0, texBox);
16
Device->SetTextureStageState(0, D3DTSS_TEXCOORDINDEX, 0);
17
Device->SetTextureStageState(0, D3DTSS_COLORARG1, D3DTA_TEXTURE); // 顏色來源-材質
18
Device->SetTextureStageState(0, D3DTSS_COLOROP, D3DTOP_SELECTARG1); // 使用當前顏色作為第一個texture stage的輸出
19
20
// 第一次描繪box
21
Box->draw(&boxWorldMatrix, 0, 0);
22
23
//使用帶alpha值得flower紋理貼圖實現第二次渲染,有alpha混合
24
Device->SetTexture(0, texAlphaFlower);
25
Device->SetTextureStageState(0, D3DTSS_TEXCOORDINDEX, 0);
26
Device->SetTextureStageState(0, D3DTSS_COLORARG1, D3DTA_TEXTURE); // 顏色來源-材質
27
Device->SetTextureStageState(0, D3DTSS_COLOROP, D3DTOP_SELECTARG1); // 使用當前顏色作為第一個texture stage的輸出
28
29
// 打開紋理混合
30
Device->SetRenderState(D3DRS_ALPHABLENDENABLE, true);
31
32
// 第一次描繪box
33
Box->draw(&boxWorldMatrix, 0, 0);
34
35
// 關閉紋理混合
36
Device->SetRenderState(D3DRS_ALPHABLENDENABLE, false);
37
}