本篇是創建游戲內核(15)的續篇,關于DirectInput使用的基礎知識請參閱使用DirectInput進行交互。
輸入內核
通過鍵盤、鼠標和游戲桿,輸入內核提供了一種手段,使玩家能夠同游戲進行交互。使用兩個簡單的類:INPUT和INPUT_DEVICE產生輸入設備。INPUT類用來初始化DirectInput,而INPUT_DEVICE類用來包含一個DirectInput設備接口對象。如果要使用多個設備,需要對每個設備使用單獨的INPUT_DEVICE對象。
使用INPUT初始化DirectInput
使用輸入系統的第一步就是初始化DirectInput,這也是INPUT類的用途。
//============================================================================
// This class encapsulates DirectInput initialize and release.
//============================================================================
class INPUT
{
protected:
HWND _hwnd;
IDirectInput8* _directinput;
public:
INPUT();
~INPUT();
IDirectInput8* get_directinput_com();
HWND get_hwnd();
BOOL init(HWND hwnd, HINSTANCE inst);
void shutdown();
};
//---------------------------------------------------------------------
// Constructor, initialize member data.
//---------------------------------------------------------------------
INPUT::INPUT()
{
// only need to clear the DirectInput interface pointer
_directinput = NULL;
}
//---------------------------------------------------------------------
// Release all DirectInput objects.
//---------------------------------------------------------------------
INPUT::~INPUT()
{
// force a shutdown
shutdown();
}
//---------------------------------------------------------------------
// Return the parent window handle.
//---------------------------------------------------------------------
HWND INPUT::get_hwnd()
{
return _hwnd;
}
//---------------------------------------------------------------------
// Return a pointer to IDirectInput8 object.
//---------------------------------------------------------------------
IDirectInput8* INPUT::get_directinput_com()
{
return _directinput;
}
//---------------------------------------------------------------------
// Create DirectInput and set window handle which pointer parent window.
//---------------------------------------------------------------------
BOOL INPUT::init(HWND hwnd, HINSTANCE inst)
{
// free DirectInput resource first
shutdown();
// record parent window handle
_hwnd = hwnd;
// create a DirectInput interface
if(FAILED(DirectInput8Create(inst, DIRECTINPUT_VERSION, IID_IDirectInput8, (void**) &_directinput, NULL)))
return FALSE;
return TRUE;
}
//---------------------------------------------------------------------
// Release all DirectInput objects.
//---------------------------------------------------------------------
void INPUT::shutdown()
{
Release_COM(_directinput);
_hwnd = NULL;
}
輸入設備和INPUT_DEVICE
INPUT_DEVICE類是輸入內核中真正起作用的類,INPUT_DEVICE類用于初始化一種特定的輸入設備(鍵盤、鼠標、游戲桿),并提供一種取得游戲使用的設備信息的方法。
//============================================================================
// This class encapsulate for all input devices (keyboard, mouse, joystick).
//============================================================================
class INPUT_DEVICE
{
public:
INPUT* m_input;
IDirectInputDevice8* m_di_device;
short m_type; // device type, can be (MOUSE, KEYNOARD, JOYSTICK)
BOOL m_use_window_mode; // whether use window mode to read mouse coordinate
char m_state[256]; // all keys information and button press information
DIMOUSESTATE* m_mouse_state; // pointer to mouse state information
DIJOYSTATE* m_joystick_state; // pointer to joystick state information
BOOL m_locks[256]; // lock flags for keyboard or button
long m_x_pos, m_y_pos; // mouse or joystick coordinate
static BOOL FAR PASCAL enum_joysticks(LPCDIDEVICEINSTANCE device_inst, LPVOID ref);
public:
INPUT_DEVICE();
~INPUT_DEVICE();
IDirectInputDevice8* get_device_com();
BOOL create(INPUT* input, short type, BOOL window_mode = TRUE);
void free();
void clear();
BOOL read();
BOOL acquire(BOOL is_active = TRUE);
BOOL get_lock(unsigned char index);
void set_lock(unsigned char index, BOOL lock_flag = TRUE);
long get_x_pos();
void set_x_pos(long x_pos);
long get_y_pos();
void set_y_pos(long y_pos);
long get_x_delta();
long get_y_delta();
// keyboard specific functions
BOOL get_key_state(unsigned char index);
void set_key_state(unsigned char index, BOOL state);
BOOL get_pure_key_state(unsigned char index);
short get_key_press(long timeout = 0);
long get_num_key_press();
long get_num_pure_key_press();
// mouse/joystick specific functions
BOOL get_button_state(unsigned char index);
BOOL set_button_state(unsigned char index, BOOL state);
BOOL get_pure_button_state(unsigned char index);
long get_num_button_press();
long get_num_pure_button_press();
};
來看看它的實現:
//---------------------------------------------------------------------
// Constructor, initialize member data.
//---------------------------------------------------------------------
INPUT_DEVICE::INPUT_DEVICE()
{
m_input = NULL;
m_di_device = NULL;
m_type = NONE; // setup device to none
m_use_window_mode = TRUE; // set windowed usage to TRUE
// point the mouse and joystick structures to the state buffer
m_mouse_state = (DIMOUSESTATE*) &m_state;
m_joystick_state = (DIJOYSTATE*) &m_state;
// clear the device variables
clear();
}
//---------------------------------------------------------------------
// Destructor, free resource.
//---------------------------------------------------------------------
INPUT_DEVICE::~INPUT_DEVICE()
{
free();
}
//---------------------------------------------------------------------
// Return the pointer to the IDirectDevice8 object
//---------------------------------------------------------------------
IDirectInputDevice8* INPUT_DEVICE::get_device_com()
{
return m_di_device;
}
//---------------------------------------------------------------------
// Create DirectInput device with specified type.
//---------------------------------------------------------------------
BOOL INPUT_DEVICE::create(INPUT* input, short type, BOOL window_mode)
{
DIDATAFORMAT* data_format;
// free a pior device
free();
// check for a valid parent INPUT class
if((m_input = input) == NULL)
return FALSE;
// create the device and rember device data format
switch(type)
{
case KEYBOARD:
if(FAILED(m_input->get_directinput_com()->CreateDevice(GUID_SysKeyboard, &m_di_device, NULL)))
return FALSE;
data_format = (DIDATAFORMAT*) &c_dfDIKeyboard;
break;
case MOUSE:
if(FAILED(m_input->get_directinput_com()->CreateDevice(GUID_SysMouse, &m_di_device, NULL)))
return FALSE;
data_format = (DIDATAFORMAT*) &c_dfDIMouse;
break;
case JOYSTICK:
if(FAILED(m_input->get_directinput_com()->EnumDevices(DI8DEVCLASS_GAMECTRL, enum_joysticks, this,
DIEDFL_ATTACHEDONLY)))
return FALSE;
if(m_di_device == NULL)
return FALSE;
data_format = (DIDATAFORMAT*) &c_dfDIJoystick;
break;
default:
return FALSE;
}
// set the windowed usage
m_use_window_mode = window_mode;
if(FAILED(m_di_device->SetDataFormat(data_format)))
return FALSE;
if(FAILED(m_di_device->SetCooperativeLevel(m_input->get_hwnd(), DISCL_FOREGROUND | DISCL_NONEXCLUSIVE)))
return FALSE;
DIPROPRANGE prop_range;
DIPROPDWORD prop_dword;
// set the special properties if it's a joystick
if(type == JOYSTICK)
{
// set the special properties of the joystick (range)
prop_range.diph.dwSize = sizeof(DIPROPRANGE);
prop_range.diph.dwHeaderSize = sizeof(DIPROPHEADER);
prop_range.diph.dwHow = DIPH_BYOFFSET;
prop_range.lMin = -1024;
prop_range.lMax = +1024;
// set x range
prop_range.diph.dwObj = DIJOFS_X;
if(FAILED(m_di_device->SetProperty(DIPROP_RANGE, &prop_range.diph)))
return FALSE;
// set y range
prop_range.diph.dwObj = DIJOFS_Y;
if(FAILED(m_di_device->SetProperty(DIPROP_RANGE, &prop_range.diph)))
return FALSE;
// set the special properties of the joystick (deadzone 12%)
prop_dword.diph.dwSize = sizeof(DIPROPDWORD);
prop_dword.diph.dwHeaderSize = sizeof(DIPROPHEADER);
prop_dword.diph.dwHow = DIPH_BYOFFSET;
prop_dword.dwData = 1200;
// set x deadzone
prop_dword.diph.dwObj = DIJOFS_X;
if(FAILED(m_di_device->SetProperty(DIPROP_DEADZONE, &prop_dword.diph)))
return FALSE;
// set y deadzone
prop_dword.diph.dwObj = DIJOFS_Y;
if(FAILED(m_di_device->SetProperty(DIPROP_DEADZONE, &prop_dword.diph)))
return FALSE;
}
// acquire the device for use
if(FAILED(m_di_device->Acquire()))
return FALSE;
// set the device type
m_type = type;
return TRUE;
}
//---------------------------------------------------------------------
// Release DirectInput device resource, reset state informations and
// lock flags.
//---------------------------------------------------------------------
void INPUT_DEVICE::free()
{
// unaquire and release the object
if(m_di_device != NULL)
{
m_di_device->Unacquire();
release_com(m_di_device);
}
// set to no device installed
m_type = NONE;
// clear state information and lock flags, reset mouse or joystick position.
clear();
}
//---------------------------------------------------------------------
// clear state information and lock flags, reset mouse or joystick
// position.
//---------------------------------------------------------------------
void INPUT_DEVICE::clear()
{
ZeroMemory(&m_state, 256);
for(short i = 0; i < 256; i++)
m_locks[i] = FALSE;
m_x_pos = m_y_pos = 0;
}
//---------------------------------------------------------------------
// Read data from DirectInput device.
//---------------------------------------------------------------------
BOOL INPUT_DEVICE::read()
{
long buffer_sizes[3] = { 256, sizeof(DIMOUSESTATE), sizeof(DIJOYSTATE) };
// make sure to have a valid IDirectInputDevice8 object
if(m_di_device == NULL)
return FALSE;
// make sure devicec type if in range
if(m_type < 1 || m_type > 3)
return FALSE;
HRESULT rv;
// loop polling and reading until succeeded or unknown error, also take care of lost-foucs problems.
while(1)
{
m_di_device->Poll();
// read in state
if(SUCCEEDED(rv = m_di_device->GetDeviceState(buffer_sizes[m_type-1], (LPVOID) &m_state)))
break;
// return on an unknown error
if(rv != DIERR_INPUTLOST && rv != DIERR_NOTACQUIRED)
return FALSE;
// reacquire an try again
if(FAILED(m_di_device->Acquire()))
return FALSE;
}
// since only the mouse coordinate are relative, you will have to deal with them now.
if(m_type == MOUSE)
{
// if windowed usage, ask windows for coordinates
if(m_use_window_mode)
{
POINT mouse_pos;
GetCursorPos(&mouse_pos);
ScreenToClient(m_input->get_hwnd(), &mouse_pos);
m_x_pos = mouse_pos.x;
m_y_pos = mouse_pos.y;
}
else
{
m_x_pos += m_mouse_state->lX;
m_y_pos += m_mouse_state->lY;
}
}
// released keys and button need to be unlocked
switch(m_type)
{
case KEYBOARD:
for(short i = 0; i < 256; i++)
{
if(! (m_state[i] & 0x80))
m_locks[i] = FALSE;
}
break;
case MOUSE:
for(short i = 0; i < 4; i++)
{
if(! (m_mouse_state->rgbButtons[i]))
m_locks[i] = FALSE;
}
break;
case JOYSTICK:
for(short i = 0; i < 32; i++)
{
if(! (m_joystick_state->rgbButtons[i]))
m_locks[i] = FALSE;
}
break;
}
return TRUE;
}
//---------------------------------------------------------------------
// Acquire or unacquire DirectInput device.
//---------------------------------------------------------------------
BOOL INPUT_DEVICE::acquire(BOOL is_active)
{
if(m_di_device == NULL)
return FALSE;
if(is_active)
m_di_device->Acquire();
else
m_di_device->Unacquire();
return TRUE;
}
//---------------------------------------------------------------------
// Get key or button lock flag with specified index.
//---------------------------------------------------------------------
BOOL INPUT_DEVICE::get_lock(unsigned char index)
{
return m_locks[index];
}
//---------------------------------------------------------------------
// Set lock flag.
//---------------------------------------------------------------------
void INPUT_DEVICE::set_lock(unsigned char index, BOOL lock_flag)
{
m_locks[index] = lock_flag;
}
//---------------------------------------------------------------------
// Get x coordinate of mouse or joystick.
//---------------------------------------------------------------------
long INPUT_DEVICE::get_x_pos()
{
// update coordinates if a joystick
if(m_type == JOYSTICK)
m_x_pos = m_joystick_state->lX;
return m_x_pos;
}
//---------------------------------------------------------------------
// Set x coordinate of mouse or joystick.
//---------------------------------------------------------------------
void INPUT_DEVICE::set_x_pos(long x_pos)
{
m_x_pos = x_pos;
}
//---------------------------------------------------------------------
// Get y coordinate of mouse or joystick.
//---------------------------------------------------------------------
long INPUT_DEVICE::get_y_pos()
{
// update coordinates if a joystick
if(m_type == JOYSTICK)
m_y_pos = m_joystick_state->lY;
return m_y_pos;
}
//---------------------------------------------------------------------
// Set y coordinate of mouse or joystick.
//---------------------------------------------------------------------
void INPUT_DEVICE::set_y_pos(long y_pos)
{
m_y_pos = y_pos;
}
//---------------------------------------------------------------------
// Get x coordinate delta of mouse or joystick.
//---------------------------------------------------------------------
long INPUT_DEVICE::get_x_delta()
{
switch(m_type)
{
case MOUSE:
return m_mouse_state->lX;
case JOYSTICK:
return m_joystick_state->lX - m_x_pos;
}
return 0;
}
//---------------------------------------------------------------------
// Get y coordinate delta of mouse or joystick.
//---------------------------------------------------------------------
long INPUT_DEVICE::get_y_delta()
{
switch(m_type)
{
case MOUSE:
return m_mouse_state->lY;
case JOYSTICK:
return m_joystick_state->lY - m_y_pos;
}
return 0;
}
//---------------------------------------------------------------------
// Check if key/button is pressed, check lock flag.
//---------------------------------------------------------------------
BOOL INPUT_DEVICE::get_key_state(unsigned char index)
{
if((m_state[index] & 0x80) && m_locks[index] == FALSE)
return TRUE;
return FALSE;
}
//---------------------------------------------------------------------
// Set key state for specified key.
//---------------------------------------------------------------------
void INPUT_DEVICE::set_key_state(unsigned char index, BOOL state)
{
m_state[index] = state;
}
//---------------------------------------------------------------------
// Check if key/button is pressed, do not check lock flag.
//---------------------------------------------------------------------
BOOL INPUT_DEVICE::get_pure_key_state(unsigned char index)
{
return (m_state[index] & 0x80) ? TRUE : FALSE;
}
//---------------------------------------------------------------------
// Get key which has been pressed and return it's ascii value.
//---------------------------------------------------------------------
short INPUT_DEVICE::get_key_press(long timeout)
{
// Retrieves the active input locale identifier (formerly called the keyboard layout) for the specified thread.
// If the idThread parameter is zero, the input locale identifier for the active thread is returned.
static HKL keyboard_layout = GetKeyboardLayout(0);
// make sure it's a keyboard and it is initialized
if((m_type != KEYBOARD) || (m_di_device == NULL))
return 0;
// calculate end time for timeout
unsigned long end_time = GetTickCount() + timeout;
unsigned char win_key_states[256], di_key_states[256];
// loop until timeout or key pressed
while(1)
{
// get windows keyboard state
GetKeyboardState(win_key_states);
// get DirectInput keyboard state
m_di_device->GetDeviceState(256, di_key_states);
// scan through looking for key presses
for(unsigned short i = 0; i < 256; i++)
{
// if one found, try to convert it.
if(di_key_states[i] & 0x80)
{
unsigned short scan_code = i;
unsigned short virtual_key, ascii_char;
// get virtual key code
if((virtual_key = MapVirtualKeyEx(scan_code, 1, keyboard_layout)))
{
// get ascii code of key and return it
if(ToAsciiEx(virtual_key, scan_code, win_key_states, &ascii_char, 0, keyboard_layout))
return ascii_char;
}
}
}
// check for timeout
if(timeout && GetTickCount() > end_time)
return 0;
}
return 0;
}
//---------------------------------------------------------------------
// Return number of key which have been pressed down, check lock flag.
//---------------------------------------------------------------------
long INPUT_DEVICE::get_num_key_press()
{
long num = 0;
for(long i = 0; i < 256; i++)
{
if((m_state[i] & 0x80) && m_locks[i] == FALSE)
num++;
}
return num;
}
//---------------------------------------------------------------------
// Return number of key which have been pressed down, ignore lock flag.
//---------------------------------------------------------------------
long INPUT_DEVICE::get_num_pure_key_press()
{
long num = 0;
for(long i = 0; i < 256; i++)
{
if(m_state[i] & 0x80)
num++;
}
return num;
}
//---------------------------------------------------------------------
// Return button state of mouse or joystick, check lock flag.
//---------------------------------------------------------------------
BOOL INPUT_DEVICE::get_button_state(unsigned char index)
{
char state = 0;
if(m_type == MOUSE)
state = m_mouse_state->rgbButtons[index];
if(m_type == JOYSTICK)
state = m_joystick_state->rgbButtons[index];
// check if key/button is pressed
if((state & 0x80) && m_locks[index] == FALSE)
return TRUE;
return FALSE;
}
//---------------------------------------------------------------------
// Set button state of mouse or joystick.
//---------------------------------------------------------------------
BOOL INPUT_DEVICE::set_button_state(unsigned char index, BOOL state)
{
if(m_type == MOUSE)
{
m_mouse_state->rgbButtons[index] = state;
return TRUE;
}
if(m_type == JOYSTICK)
{
m_joystick_state->rgbButtons[index] = state;
return TRUE;
}
return FALSE;
}
//---------------------------------------------------------------------
// Return button state of mouse or joystick, ignore lock flag.
//---------------------------------------------------------------------
BOOL INPUT_DEVICE::get_pure_button_state(unsigned char index)
{
if(m_type == MOUSE)
return m_mouse_state->rgbButtons[index];
if(m_type == JOYSTICK)
return m_joystick_state->rgbButtons[index];
return FALSE;
}
//---------------------------------------------------------------------
// Return number of buttons of mouse and joystick which have been pressed
// down, check lock flag.
//---------------------------------------------------------------------
long INPUT_DEVICE::get_num_button_press()
{
long num = 0;
if(m_type == MOUSE)
{
for(long i = 0; i < 4; i++)
{
if((m_mouse_state->rgbButtons[i] & 0x80) && m_locks[i] == FALSE)
num++;
}
}
else if(m_type == JOYSTICK)
{
for(long i = 0; i < 32; i++)
{
if((m_joystick_state->rgbButtons[i] & 0x80) && m_locks[i] == FALSE)
num++;
}
}
return num;
}
//---------------------------------------------------------------------
// Return number of buttons of mouse and joystick which have been pressed
// down, ignore lock flag.
//---------------------------------------------------------------------
long INPUT_DEVICE::get_num_pure_button_press()
{
long num = 0;
if(m_type == MOUSE)
{
for(long i = 0; i < 4; i++)
{
if(m_mouse_state->rgbButtons[i] & 0x80)
num++;
}
}
else if(m_type == JOYSTICK)
{
for(long i = 0; i < 32; i++)
{
if(m_joystick_state->rgbButtons[i] & 0x80)
num++;
}
}
return num;
}
//---------------------------------------------------------------------
// Enumerate first usable joystick.
//---------------------------------------------------------------------
BOOL FAR PASCAL INPUT_DEVICE::enum_joysticks(LPCDIDEVICEINSTANCE device_inst, LPVOID ref)
{
INPUT_DEVICE* input_device = (INPUT_DEVICE*) ref;
// stop enumeration if no parent INPUT_DEVICE pointer
if(input_device == NULL)
return DIENUM_STOP;
IDirectInput8* di = input_device->m_input->get_directinput_com();
// try to create a joystick interface
if(FAILED(di->CreateDevice(device_inst->guidInstance, &input_device->m_di_device, NULL)))
return DIENUM_CONTINUE;
// all done, stop enumeration.
return DIENUM_STOP;
}
INPUT_DEVICE類將所有的設備(鍵盤、鼠標、游戲桿)囊括在一個完美的包中。通過調用INPUT_DEVICE::create函數并傳遞一個預初始化的INPUT類對象,就可以使用INPUT_DEVICE類對象了。還需要給type參數設置一個合適的值(KEYBOARD、MOUSE或JOYSTICK),以告訴類要使用哪種設備。最后,還需要告訴類如何讀取鼠標狀態,包括使用DirectInput的設備讀取函數或windows的設備讀取函數兩種方式。將window_mode參數設置為TRUE會強制類對象使用windows的設備讀取函數,而使用FALSE就會強制類對象使用
DirectInput的設備讀取函數。如果計劃使用窗口應用程序(或希望windows光標可見),就要確將window_mode參數設置為TRUE。
使用INPUT_DEVICE::read函數可以讀取正在使用的設備的當前狀態。
下面編寫測試代碼:
點擊下載源碼和工程
/*****************************************************************************
PURPOSE:
Test for class INPUT and INPUT_DEVICE.
*****************************************************************************/
#include "Core_Global.h"
class APP : public APPLICATION
{
private:
GRAPHICS _graphics;
INPUT _input;
INPUT_DEVICE _keyboard;
INPUT_DEVICE _mouse;
INPUT_DEVICE _joystick;
LONG _joystick_x, _joystick_y;
public:
APP()
{
_joystick_x = _joystick_y = 0;
}
BOOL init();
BOOL frame();
};
BOOL APP::init()
{
// initialie graphics
if(! _graphics.init())
return FALSE;
// get screen width and height
int screen_width = GetSystemMetrics(SM_CXSCREEN);
int screen_height = GetSystemMetrics(SM_CYSCREEN);
// set display mode for graphics
if(! _graphics.set_mode(get_hwnd(), TRUE, FALSE, screen_width, screen_height, 16))
return FALSE;
// initialize DirectInput
_input.init(get_hwnd(), get_inst());
// create keyboad, mouse, joystick
_keyboard.create(&_input, KEYBOARD);
_mouse.create(&_input, MOUSE, FALSE);
_joystick.create(&_input, JOYSTICK, FALSE);
return TRUE;
}
BOOL APP::frame()
{
// clear display with specified color
_graphics.clear_display(D3DCOLOR_RGBA(0, 0, 0, 255));
// read current state information
_keyboard.read();
_mouse.read();
_joystick.read();
// begin scene
if(_graphics.begin_scene())
{
// if key 'ESC' has been pressed, show message box.
if(_keyboard.get_key_state(KEY_ESC))
{
// lock this key
_keyboard.set_lock(KEY_ESC, TRUE);
// show information
MessageBox(get_hwnd(), "ESCAPE", "Key Pressed!", MB_OK);
}
char buffer[200];
// if left button of mouse is pressed, show message box.
if(_mouse.get_pure_button_state(MOUSE_LBUTTON))
{
sprintf(buffer, "%ld, %ld", _mouse.get_x_pos(), _mouse.get_y_pos());
MessageBox(get_hwnd(), buffer, "Mouse Coordinate!", MB_OK);
}
if(_joystick.get_button_state(JOYSTICK_BUTTON0))
{
MessageBox(get_hwnd(), "Joystick button0 is pressed down!", "Joystick", MB_OK);
_joystick.set_lock(JOYSTICK_BUTTON0, TRUE);
}
if(_joystick_x != _joystick.get_x_pos() || _joystick_y != _joystick.get_y_pos())
{
sprintf(buffer, "%ld, %ld", _joystick.get_x_pos(), _joystick.get_y_pos());
MessageBox(get_hwnd(), buffer, "Joystick Coordinate!", MB_OK);
_joystick_x = _joystick.m_x_pos;
_joystick_y = _joystick.m_y_pos;
}
// end the scene
_graphics.end_scene();
}
// display video buffer
_graphics.display();
return TRUE;
}
int PASCAL WinMain(HINSTANCE inst, HINSTANCE, LPSTR cmd_line, int cmd_show)
{
APP app;
return app.run();
}
程序截圖:
按下游戲桿按鍵0:
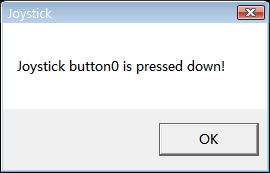
獲得游戲桿的坐標:
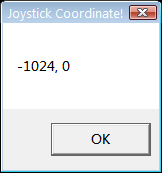
按下鍵盤按鍵ESC:
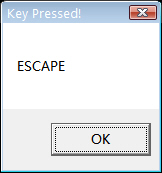
按下鼠標左鍵:
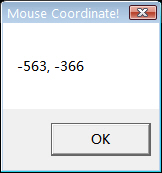