本篇是使用DirectInput進行交互(2)的續篇。
使用鼠標玩游戲
鼠標的工作原理理解起來并不難,在最底層,鼠標通知系統它要移動到某個方向,每次移動一個記號,驅動程序讀取這個數據后,將記號轉化為相對移動值。
在通常的應用程序中,windows得到鼠標的移動并通過消息處理函數將移動作為消息報告給用戶。使用消息處理函數有時速度會非常慢,因為傳遞給消息處理函數的每個消息要被插入到隊列中,這樣消息就只會按照他們加入到隊列中的順序被處理。要加快接收以及處理鼠標輸入的過程,就必須直接同鼠標的驅動程序進行交互,而不采用windows消息處理函數。
不論采用哪種移動方式接收鼠標移動,都要從跟蹤鼠標在屏幕上的坐標開始,可以選擇追蹤絕對鼠標坐標或相對鼠標坐標。絕對表示當前的鼠標位置都是基于某個固定點(通常是屏幕的左上角)。
如下圖所示,通過鼠標距屏幕左上角的像素數量來衡量鼠標的絕對坐標。
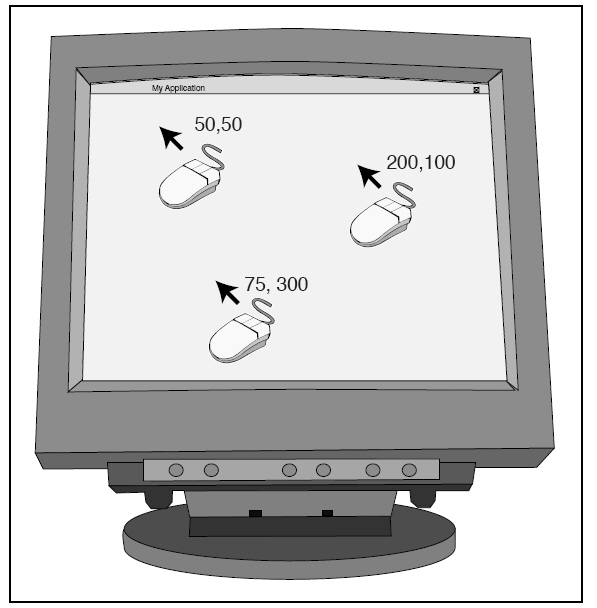
相對指的是從上個已知位置到當前位置所發生的移動量,上個位置可能位于左邊、右邊、上邊或下邊。
使用DirectInput處理鼠標
除了指定的是鼠標標識符以及鼠標數據格式外,初始化鼠標就和初始化鍵盤幾乎完全相同。
//--------------------------------------------------------------------------------
// Initialize mouse interface, return a mouse interface pointer.
//--------------------------------------------------------------------------------
IDirectInputDevice8* Init_Mouse(HWND hwnd, IDirectInput8* directinput)
{
IDirectInputDevice8* directinput_device;
// create the device object
if(FAILED(directinput->CreateDevice(GUID_SysMouse, &directinput_device, NULL)))
return NULL;
// set the data format
if(FAILED(directinput_device->SetDataFormat(&c_dfDIMouse)))
{
directinput_device->Release();
return NULL;
}
// set the coooperative mode
if(FAILED(directinput_device->SetCooperativeLevel(hwnd, DISCL_FOREGROUND | DISCL_NONEXCLUSIVE)))
{
directinput_device->Release();
return NULL;
}
// acquire the device for use
if(FAILED(directinput_device->Acquire()))
{
directinput_device->Release();
return NULL;
}
// everything well, so return a vaild pointer.
return directinput_device;
}
要調用DirectInputDevice8::GetDeviceState,使用諸如相對移動和按鍵狀態等鼠標的相關信息來填充 DIMOUSESTATE結構體。
DIMOUSESTATE結構體的定義如下:
Describes the state of a mouse device that has up to
four buttons, or another device that is being accessed as if it were a mouse
device. This structure is used with the IDirectInputDevice8::GetDeviceState
method.
typedef struct DIMOUSESTATE {
LONG lX;
LONG lY;
LONG lZ;
BYTE rgbButtons[4];
} DIMOUSESTATE, *LPDIMOUSESTATE;
Members
- lX
- X-axis.
- lY
- Y-axis.
- lZ
- Z-axis, typically a wheel. If the mouse does not have a z-axis, the
value is 0.
- rgbButtons
- Array of buttons. The high-order bit of the byte is set if the
corresponding button is down.
Remarks
You must prepare the device for mouse-style access by calling the
IDirectInputDevice8::SetDataFormat method, passing the c_dfDIMouse global data
format variable.
The mouse is a relative-axis device, so the absolute axis positions for mouse
axes are accumulated relative motion. Therefore, the value of the absolute axis
position is not meaningful except in comparison with other absolute axis
positions.
If an axis is in relative mode, the appropriate member contains the change in
position. If it is in absolute mode, the member contains the absolute axis
position.
點擊下載源碼和工程
完整源碼示例:
/***************************************************************************************
PURPOSE:
Mouse device Demo
***************************************************************************************/
#define DIRECTINPUT_VERSION 0x0800
#include <windows.h>
#include <stdio.h>
#include <dinput.h>
#include "resource.h"
#pragma comment(lib, "dxguid.lib")
#pragma comment(lib, "dinput8.lib")
#pragma warning(disable : 4996)
#define Safe_Release(p) if((p)) (p)->Release();
// window handles, class and caption text.
HWND g_hwnd;
char g_class_name[] = "MouseClass";
IDirectInput8* g_directinput; // directinput component
IDirectInputDevice8* g_directinput_device; // mouse device
//--------------------------------------------------------------------------------
// Window procedure.
//--------------------------------------------------------------------------------
long WINAPI Window_Proc(HWND hwnd, UINT msg, WPARAM wParam, LPARAM lParam)
{
switch(msg)
{
case WM_DESTROY:
PostQuitMessage(0);
return 0;
}
return (long) DefWindowProc(hwnd, msg, wParam, lParam);
}
//--------------------------------------------------------------------------------
// Initialize mouse interface, return a mouse interface pointer.
//--------------------------------------------------------------------------------
IDirectInputDevice8* Init_Mouse(HWND hwnd, IDirectInput8* directinput)
{
IDirectInputDevice8* directinput_device;
// create the device object
if(FAILED(directinput->CreateDevice(GUID_SysMouse, &directinput_device, NULL)))
return NULL;
// set the data format
if(FAILED(directinput_device->SetDataFormat(&c_dfDIMouse)))
{
directinput_device->Release();
return NULL;
}
// set the coooperative mode
if(FAILED(directinput_device->SetCooperativeLevel(hwnd, DISCL_FOREGROUND | DISCL_NONEXCLUSIVE)))
{
directinput_device->Release();
return NULL;
}
// acquire the device for use
if(FAILED(directinput_device->Acquire()))
{
directinput_device->Release();
return NULL;
}
// everything well, so return a vaild pointer.
return directinput_device;
}
//--------------------------------------------------------------------------------
// Read mouse buffer.
//--------------------------------------------------------------------------------
BOOL Read_Device(IDirectInputDevice8* directinput_device, void* buffer, long buffer_size)
{
HRESULT rv;
while(1)
{
// poll device
g_directinput_device->Poll();
// read in state
if(SUCCEEDED(rv = g_directinput_device->GetDeviceState(buffer_size, buffer)))
break;
// return when an unknown error
if(rv != DIERR_INPUTLOST || rv != DIERR_NOTACQUIRED)
return FALSE;
// re-acquire and try again
if(FAILED(g_directinput_device->Acquire()))
return FALSE;
}
return TRUE;
}
//--------------------------------------------------------------------------------
// Main function, routine entry.
//--------------------------------------------------------------------------------
int WINAPI WinMain(HINSTANCE inst, HINSTANCE, LPSTR cmd_line, int cmd_show)
{
WNDCLASS win_class;
MSG msg;
DIMOUSESTATE mouse_state = {0};
char text[256];
long x_pos = 0, y_pos = 0;
// create window class and register it
win_class.style = CS_HREDRAW | CS_VREDRAW;
win_class.lpfnWndProc = Window_Proc;
win_class.cbClsExtra = 0;
win_class.cbWndExtra = DLGWINDOWEXTRA;
win_class.hInstance = inst;
win_class.hIcon = LoadIcon(inst, IDI_APPLICATION);
win_class.hCursor = LoadCursor(NULL, IDC_ARROW);
win_class.hbrBackground = (HBRUSH) (COLOR_BTNFACE + 1);
win_class.lpszMenuName = NULL;
win_class.lpszClassName = g_class_name;
if(! RegisterClass(&win_class))
return FALSE;
// create the main window
g_hwnd = CreateDialog(inst, MAKEINTRESOURCE(IDD_MOUSE), 0, NULL);
ShowWindow(g_hwnd, cmd_show);
UpdateWindow(g_hwnd);
// initialize directinput and get keyboard device
DirectInput8Create(inst, DIRECTINPUT_VERSION, IID_IDirectInput8, (void **) &g_directinput, NULL);
// initialize mouse
g_directinput_device = Init_Mouse(g_hwnd, g_directinput);
// start message pump, waiting for signal to quit.
ZeroMemory(&msg, sizeof(MSG));
while(msg.message != WM_QUIT)
{
if(PeekMessage(&msg, NULL, 0, 0, PM_REMOVE))
{
TranslateMessage(&msg);
DispatchMessage(&msg);
}
// read in mouse and display coordinates
Read_Device(g_directinput_device, &mouse_state, sizeof(DIMOUSESTATE));
x_pos += mouse_state.lX;
y_pos += mouse_state.lY;
if(mouse_state.lX != 0 || mouse_state.lY != 0)
{
sprintf(text, "%ld, %ld", x_pos, y_pos);
SetWindowText(GetDlgItem(g_hwnd, IDC_COORDINATES), text);
}
}
// release directinput objects
g_directinput_device->Unacquire();
g_directinput_device->Release();
g_directinput->Release();
UnregisterClass(g_class_name, inst);
return (int) msg.wParam;
}
運行截圖:
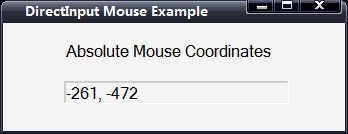
閱讀下篇:
使用DirectInput進行交互(4)