作用:
運用共享技術有效地支持大量細粒度的對象。
UML結構圖:
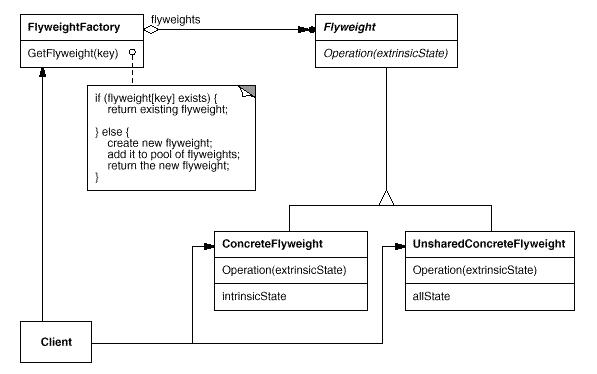
解析:
Flyweight模式在大量使用一些可以被共享的對象的時候經常使用.比如,在QQ聊天的時候很多時候你懶得回復又不得不回復的時候,一般會用一些客套的話語敷衍別人,如"呵呵","好的"等等之類的,這些簡單的答復其實每個人都是提前定義好的,在使用的時候才調用出來.Flyweight就是基于解決這種問題的思路而產生的,當需要一個可以在其它地方共享使用的對象的時候,先去查詢是否已經存在了同樣的對象,如果沒有就生成之有的話就直接使用.因此,Flyweight模式和Factory模式也經常混用.
實現:
需要說明的是下面的實現僅僅實現了對可共享對象的使用,非可共享對象的使用沒有列出,因為這個不是Flyweight模式的重點.這里的實現要點是采用一個list鏈表來保存這些可以被共享的對象,需要使用的時候就到鏈表中查詢是不是已經存在了,如果不存在就初始化一個,然后返回這個對象的指針.
1)Flyweight.h

/**//********************************************************************
????created:????2006/07/26
????filename:?????FlyWeight.h
????author:????????李創
????????????????http://www.shnenglu.com/converse/

????purpose:????FlyWeight模式的演示代碼
*********************************************************************/

#ifndef?FLYWEIGHT_H
#define?FLYWEIGHT_H

#include?<string>
#include?<list>

typedef?std::string?STATE;

class?Flyweight


{
public:

????virtual?~Flyweight()
{}

????STATE?GetIntrinsicState();
????virtual?void?Operation(STATE&?ExtrinsicState)?=?0;

protected:
????Flyweight(const?STATE&?state)?
????????:m_State(state)

????
{
????}

private:
????STATE?m_State;
};

class?FlyweightFactory


{
public:

????FlyweightFactory()
{}
????~FlyweightFactory();

????Flyweight*?GetFlyweight(const?STATE&?key);

private:
????std::list<Flyweight*>????m_listFlyweight;
};

class?ConcreateFlyweight
????:?public?Flyweight


{
public:
????ConcreateFlyweight(const?STATE&?state)
????????:?Flyweight(state)

????
{
????}

????virtual?~ConcreateFlyweight()
{}

????virtual?void?Operation(STATE&?ExtrinsicState);
};

#endif

2)Flyweight.cpp

/**//********************************************************************
????created:????2006/07/26
????filename:?????FlyWeight.cpp
????author:????????李創
????????????????http://www.shnenglu.com/converse/

????purpose:????FlyWeight模式的演示代碼
*********************************************************************/

#include?"FlyWeight.h"
#include?<iostream>

inline?STATE?Flyweight::GetIntrinsicState()


{
????return?m_State;
}

FlyweightFactory::~FlyweightFactory()


{
????std::list<Flyweight*>::iterator?iter1,?iter2,?temp;

????for?(iter1?=?m_listFlyweight.begin(),?iter2?=?m_listFlyweight.end();
????????iter1?!=?iter2;
????????)

????
{
????????temp?=?iter1;
????????++iter1;
????????delete?(*temp);
????}

????m_listFlyweight.clear();
}

Flyweight*?FlyweightFactory::GetFlyweight(const?STATE&?key)


{
????std::list<Flyweight*>::iterator?iter1,?iter2;

????for?(iter1?=?m_listFlyweight.begin(),?iter2?=?m_listFlyweight.end();
?????????iter1?!=?iter2;
?????????++iter1)

????
{
????????if?((*iter1)->GetIntrinsicState()?==?key)

????????
{
????????????std::cout?<<?"The?Flyweight:"?<<?key?<<?"?already?exits"<<?std::endl;
????????????return?(*iter1);
????????}
????}

????std::cout?<<?"Creating?a?new?Flyweight:"?<<?key?<<?std::endl;
????Flyweight*?flyweight?=?new?ConcreateFlyweight(key);
????m_listFlyweight.push_back(flyweight);
}

void?ConcreateFlyweight::Operation(STATE&?ExtrinsicState)


{

}

3)Main.cpp

/**//********************************************************************
????created:????2006/07/26
????filename:?????Main.cpp
????author:????????李創
????????????????http://www.shnenglu.com/converse/

????purpose:????FlyWeight模式的測試代碼
*********************************************************************/

#include?"FlyWeight.h"

int?main()


{
????FlyweightFactory?flyweightfactory;
????flyweightfactory.GetFlyweight("hello");
????flyweightfactory.GetFlyweight("world");
????flyweightfactory.GetFlyweight("hello");

????system("pause");
????return?0;
}
