作用:
允許一個對象在其內(nèi)部狀態(tài)改變時改變它的行為.
UML結構圖:
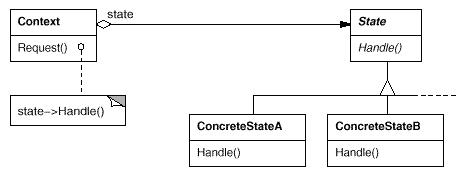
解析:
State模式主要解決的是在開發(fā)中時常遇到的根據(jù)不同的狀態(tài)需要進行不同的處理操作的問題,而這樣的問題,大部分人是采用switch-case語句進行處理的,這樣會造成一個問題:分支過多,而且如果加入一個新的狀態(tài)就需要對原來的代碼進行編譯.State模式采用了對這些不同的狀態(tài)進行封裝的方式處理這類問題,當狀態(tài)改變的時候進行處理然后再切換到另一種狀態(tài),也就是說把狀態(tài)的切換責任交給了具體的狀態(tài)類去負責.同時,State模式和Strategy模式在圖示上有很多相似的地方,需要說明的是兩者的思想都是一致的,只不過封裝的東西不同:State模式封裝的是不同的狀態(tài),而Stategy模式封裝的是不同的算法.
實現(xiàn):
1)State.h

/**//********************************************************************
????created:????2006/08/05
????filename:?????State.h
????author:????????李創(chuàng)
????????????????http://www.shnenglu.com/converse/

????purpose:????State模式的演示代碼
*********************************************************************/

#ifndef?STATE_H
#define?STATE_H

class?State;

class?Context


{
public:
????Context(State*?pState);
????~Context();
????void?Request();
????void?ChangeState(State?*pState);

private:
????State?*m_pState;
};

class?State


{
public:

????virtual?~State()
{}

????virtual?void?Handle(Context*?pContext)?=?0;
};

class?ConcreateStateA
????:?public?State


{
public:
????void?Handle(Context*?pContext);
};

class?ConcreateStateB
????:?public?State


{
public:
????void?Handle(Context*?pContext);
};

#endif

2)State.cpp

/**//********************************************************************
????created:????2006/08/05
????filename:?????State.cpp
????author:????????李創(chuàng)
????????????????http://www.shnenglu.com/converse/

????purpose:????State模式的演示代碼
*********************************************************************/

#include?"State.h"
#include?<iostream>

Context::Context(State*?pState)
????:?m_pState(pState)


{

}

Context::~Context()


{
????delete?m_pState;
????m_pState?=?NULL;
}

void?Context::Request()


{
????if?(NULL?!=?m_pState)

????
{
????????m_pState->Handle(this);
????}
}

void?Context::ChangeState(State?*pState)


{
????if?(NULL?!=?m_pState)

????
{
????????delete?m_pState;
????????m_pState?=?NULL;
????}
????
????m_pState?=?pState;
}

void?ConcreateStateA::Handle(Context*?pContext)


{
????std::cout?<<?"Handle?by?ConcreateStateA\n";
????
????if?(NULL?!=?pContext)

????
{
????????pContext->ChangeState(new?ConcreateStateB());
????}
}

void?ConcreateStateB::Handle(Context*?pContext)


{
????std::cout?<<?"Handle?by?ConcreateStateB\n";

????if?(NULL?!=?pContext)

????
{
????????pContext->ChangeState(new?ConcreateStateA());
????}
}

3)Main.cpp

/**//********************************************************************
????created:????2006/08/05
????filename:?????Main.cpp
????author:????????李創(chuàng)
????????????????http://www.shnenglu.com/converse/

????purpose:????State模式的測試代碼
*********************************************************************/

#include?"State.h"

int?main()


{
????State?*pState?=?new?ConcreateStateA();
????Context?*pContext?=?new?Context(pState);
????pContext->Request();
????pContext->Request();
????pContext->Request();

????delete?pContext;

????return?0;
}
