做網(wǎng)絡(luò)模塊的時(shí)候經(jīng)常會(huì)遇到有關(guān)網(wǎng)址的處理.一般大致的情形是3種:
1.得到的是域名,如:www.3322.org
2.得到的是實(shí)際的IP地址,如:61.160.235.203
3.得到的是經(jīng)過(guò)inet_addr處理過(guò)的IP,為unsigned long(DWORD)
一.那么如果是給出點(diǎn)分制的IP要轉(zhuǎn)為DWORD型是如何轉(zhuǎn)化呢?這個(gè)其實(shí)最簡(jiǎn)單,有專門(mén)的函數(shù)專門(mén)處理此事
unsigned long dwIP = inet_addr("222.212.12.77");
printf("IP(%s)->DWORD(%lu)\n");
//output
IP(222.212.12.77)->DWORD(1292686558)
二.第一種情況的逆轉(zhuǎn)化
#include <stdio.h>
#include <stdlib.h>
#include <sys/select.h>
#include <netdb.h>
#include <sys/socket.h>
#include <netinet/in.h>
#include <arpa/inet.h>
#include <errno.h>
#include <string.h>
int main(int argc, char *argv[])
{
struct in_addr net;
char tmp[16] = {0};
if(argc != 3)
{
printf("You MUST enter 3 varibal.No.1:func name No.2:case.No.3:ip(string or DWORD)\n");
return 0;
}
if(strcmp("1", argv[1]) == 0)
{
char* ip_string;
ip_string = argv[2];
unsigned long dword = inet_addr(ip_string);
printf("IP(%s)-->DWORD(%lu)\n", ip_string, dword);
}
else if(strcmp("2", argv[1]) == 0)
{
net.s_addr = (unsigned long)atol(argv[2]);
strcpy(tmp, inet_ntoa(net));
printf("DWORD(%s)-->IP(%s)\n",argv[2], tmp);
}
return 0;
}
這里給出一個(gè)點(diǎn)分制IP和DWORD相互轉(zhuǎn)化的程序
三.如果給出的是域名而想得到點(diǎn)分制的IP呢?
這里給出一個(gè)接口,支持輸入的類型是點(diǎn)分制和域名2中類型,返回的是DWORD型的IP
有一點(diǎn)要聲明的是gethostbyname這個(gè)函數(shù)必須在網(wǎng)絡(luò)連通的情況下才能正確完成域名的解析,你想,連個(gè)網(wǎng)都不通,它怎么解析?
#include <stdio.h>
#include <stdlib.h>
#include <sys/select.h>
#include <netdb.h>
#include <sys/socket.h>
#include <netinet/in.h>
#include <arpa/inet.h>
#include <errno.h>
#include <string.h>
#define DWORD unsigned long
DWORD platform_domain2ip(const char *szDomain, char *szDotNumIP)
{
char szAddr[32] = {0};
struct hostent *pHost;
printf("input domain name(%s)\n", szDomain);
if((pHost = gethostbyname(szDomain)) == NULL)
{
printf("can not parse domain\n");
return -1;
}
printf("HostName :%s\n",pHost->h_name);
strcpy(szAddr, inet_ntoa(*((struct in_addr *)pHost->h_addr)));
printf("IP Address :%s\n", szAddr);
strcpy(szDotNumIP, szAddr);
return inet_addr(szAddr);
}
int main(int argc, char *argv[])
{
DWORD dwip;
char *ip = malloc(32);
//dwip = platform_domain2ip("www.3322.org", ip);
dwip = platform_domain2ip("61.160.235.203", ip);
printf("ip 1 (%s) 2 dw(%lu)\n", ip, dwip);
return 0;
}
//可以將main的注冊(cè)分別打開(kāi)來(lái)判斷下結(jié)果是否正確,這里給出運(yùn)行的結(jié)果,有圖有真相
編譯的命令再說(shuō)下吧,怕有人不知道
gcc gethost.c –Wall –o gethost//在linux下
arm-hismall-linux-gcc gethost.c –Wall –o gethost//嵌入式環(huán)境下
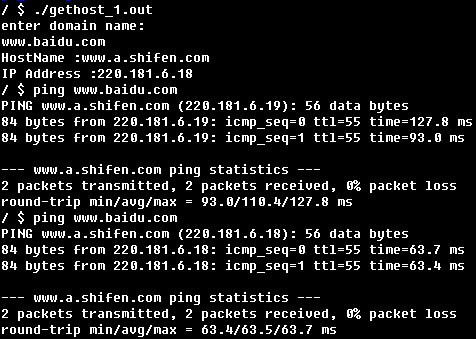
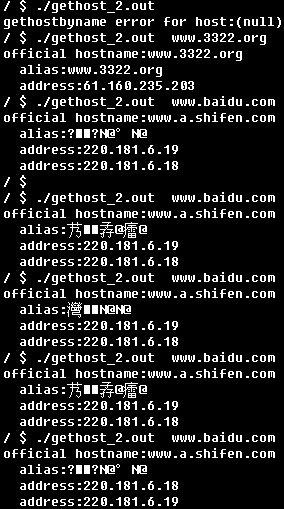
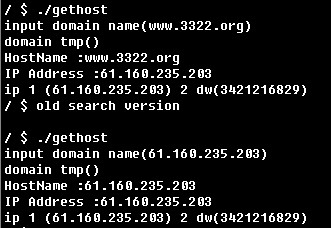
此接口已經(jīng)在我的工程中使用,在平臺(tái)IP的解析和3322的解析中得到了應(yīng)用,所以是穩(wěn)定可行的.
這3中IP的轉(zhuǎn)化都了解了的話,那么網(wǎng)絡(luò)編程不就掃除了一個(gè)大石頭嗎?呵呵,大家功能進(jìn)步
網(wǎng)上比較流行的gethostbyname的例子如下,受到了啟發(fā)
#include <stdio.h>
#include <stdlib.h>
#include <sys/select.h>
#include <netdb.h>
#include <sys/socket.h>
#include <netinet/in.h>
#include <arpa/inet.h>
#include <errno.h>
#include <string.h>
int main(int argc, char **argv)
{
char *ptr,**pptr;
struct hostent *hptr;
char str[32];
/* 取得命令后第一個(gè)參數(shù),即要解析的域名或主機(jī)名 */
ptr = argv[1];
/* 調(diào)用gethostbyname()。調(diào)用結(jié)果都存在hptr中 */
if((hptr = gethostbyname(ptr)) == NULL)
{
printf("gethostbyname error for host:%s\n", ptr);
return 1; /* 如果調(diào)用gethostbyname發(fā)生錯(cuò)誤,返回1 */
}
printf("official hostname:%s\n",hptr->h_name);
/* 主機(jī)可能有多個(gè)別名,將所有別名分別打出來(lái) */
for(pptr = hptr->h_aliases; *pptr != NULL; pptr++)
printf(" alias:%s\n",*pptr);
/* 根據(jù)地址類型,將地址打出來(lái) */
switch(hptr->h_addrtype)
{
case AF_INET:
case AF_INET6:
pptr=hptr->h_addr_list;
/* 將剛才得到的所有地址都打出來(lái)。其中調(diào)用了inet_ntop()函數(shù)*/
for(;*pptr!=NULL;pptr++)
printf(" address:%s\n", inet_ntop(hptr->h_addrtype,*pptr, str, sizeof(str)));
break;
default:
printf("unknown address type\n");
break;
}
return 0;
}