Mesh的頂點和索引能夠被重組以便能更有效的渲染mesh。當我們這樣做時,我們說我們優(yōu)化了一個mesh。我們可以使用下面的方法來進行優(yōu)化:
HRESULT
ID3DXMesh::OptimizeInplace(
DWORD Flags,
CONST DWORD*
pAdjacencyIn,
DWORD*
pAdjacencyOut,
DWORD* pFaceRemap,
LPD3DXBUFFER*
ppVertexRemap
);
|
Flags
— 表示執(zhí)行什么類型的優(yōu)化方法。它可以是下面的一個或幾個的組合:
D3DXMESHOPT_COMPACT
— 從mesh中移除沒有用的頂點和索引項。
D3DXMESHOPT_ATTRSORT
— 根據(jù)屬性給三角形排序并調整屬性表,這將使DrawSubset執(zhí)行更有效。
D3DXMESHOPT_VERTEXCACHE
— 增加頂點緩存的命中率。
D3DXMESHOPT_STRIPREORDER
— 重組頂點索引使三角帶盡可能的長。
D3DXMESHOPT_IGNOREVERTS
— 只優(yōu)化索引信息,忽略頂點信息。
注意:D3DXMESHOPT_VERTEXCACHE和D3DXMESHOPT_STRIPREORDER不能同時使用。
pAdjacencyIn
— 指向沒有優(yōu)化的mesh的鄰接數(shù)組。
pAdjacencyOut
— 指向一個DWORD數(shù)組,它被用來填充優(yōu)化好了的mesh鄰接信息。該數(shù)組必須有ID3DXMesh::GetNumFaces()
* 3個元素。如果不需要該信息,可以將其設置為0。
pFaceRemap
—指向一個DWORD數(shù)組,它被用來填充面重影射信息。該數(shù)組必須不小于ID3DXMesh::GetNumFaces()。當一個mesh被優(yōu)化時,由索引緩存定義的面可能被移動;也就是說,在pFaceRemap中的第i項表示第i個原始面被移動到的面索引值。如果不需要該信息,可以將其設置為0。
ppVertexRemap
— 指向ID3DXBuffer指針的地址,它被用來填充頂點重影射信息。這個緩存應該包含ID3DXMesh::GetNumVertices()個頂點。當一個mesh被優(yōu)化后,頂點可能被移動。頂點重影射信息用來說明原來的頂點被移動到新位置;也就是說,在ppVertexRemap中的第i項表示原來的第i個頂點的新位置。如果不需要該信息,可以將其設置為0。
例子:
// Get the
adjacency info of the non-optimized mesh.
DWORD
adjacencyInfo[Mesh->GetNumFaces() * 3];
Mesh->GenerateAdjacency(0.0f, adjacencyInfo);
// Array to
hold optimized adjacency info.
DWORD
optimizedAdjacencyInfo[Mesh->GetNumFaces() * 3];
Mesh->OptimizeInplace(D3DXMESHOPT_ATTRSORT | D3DXMESHOPT_COMPACT |
D3DXMESHOPT_VERTEXCACHE,
adjacencyInfo,
optimizedAdjacencyInfo, 0, 0);
|
一個更簡單的方法是Optimize方法,它輸出一個優(yōu)化的mesh,而不是在原來mesh的基礎上進行優(yōu)化:
Generates a new mesh with reordered faces and vertices
to optimize drawing performance.
HRESULT Optimize(
DWORD Flags,
CONST DWORD * pAdjacencyIn,
DWORD * pAdjacencyOut,
DWORD * pFaceRemap,
LPD3DXBUFFER * ppVertexRemap,
LPD3DXMESH * ppOptMesh
);
Parameters
- Flags
- [in] Specifies the type of optimization to
perform. This parameter can be set to a combination of one or more flags
from D3DXMESHOPT and D3DXMESH (except D3DXMESH_32BIT, D3DXMESH_IB_WRITEONLY,
and D3DXMESH_WRITEONLY).
- pAdjacencyIn
- [in] Pointer to an array of three DWORDs per face
that specifies the three neighbors for each face in the source mesh. If the
edge has no adjacent faces, the value is 0xffffffff. See Remarks.
- pAdjacencyOut
- [in, out] Pointer to an array of three DWORDs per
face that specifies the three neighbors for each face in the optimized mesh.
If the edge has no adjacent faces, the value is 0xffffffff.
- pFaceRemap
- [in, out] An array of DWORDs, one per face, that
identifies the original mesh face that corresponds to each face in the
optimized mesh. If the value supplied for this argument is NULL, face remap
data is not returned.
- ppVertexRemap
- [out] Address of a pointer to an ID3DXBuffer
interface, which contains a DWORD for each vertex that specifies how the new
vertices map to the old vertices. This remap is useful if you need to alter
external data based on the new vertex mapping.
- ppOptMesh
- [out] Address of a pointer to an ID3DXMesh
interface, representing the optimized mesh.
Return Values
If the method succeeds, the return value is D3D_OK. If
the method fails, the return value can be one of the following:
D3DERR_INVALIDCALL, E_OUTOFMEMORY.
Remarks
This method generates a new mesh. Before running
Optimize, an application must generate an adjacency buffer by calling
ID3DXBaseMesh::GenerateAdjacency. The adjacency buffer contains adjacency data,
such as a list of edges and the faces that are adjacent to each other.
This method is very similar to the
ID3DXBaseMesh::CloneMesh method, except that it can perform optimization while
generating the new clone of the mesh. The output mesh inherits all of the
creation parameters of the input mesh.
D3DXMESHOPT
Specifies the type of mesh optimization to be
performed.
typedef enum D3DXMESHOPT
{
D3DXMESHOPT_COMPACT = 0x01000000,
D3DXMESHOPT_ATTRSORT = 0x02000000,
D3DXMESHOPT_VERTEXCACHE = 0x04000000,
D3DXMESHOPT_STRIPREORDER = 0x08000000,
D3DXMESHOPT_IGNOREVERTS = 0x10000000,
D3DXMESHOPT_DONOTSPLIT = 0x20000000,
D3DXMESHOPT_DEVICEINDEPENDENT = 0x40000000,
} D3DXMESHOPT, *LPD3DXMESHOPT;
Constants
- D3DXMESHOPT_COMPACT
- Reorders faces to remove unused vertices and
faces.
- D3DXMESHOPT_ATTRSORT
- Reorders faces to optimize for fewer attribute
bundle state changes and enhanced ID3DXBaseMesh::DrawSubset performance.
- D3DXMESHOPT_VERTEXCACHE
- Reorders faces to increase the cache hit rate of
vertex caches.
- D3DXMESHOPT_STRIPREORDER
- Reorders faces to maximize length of adjacent
triangles.
- D3DXMESHOPT_IGNOREVERTS
- Optimize the faces only; do not optimize the
vertices.
- D3DXMESHOPT_DONOTSPLIT
- While attribute sorting, do not split vertices
that are shared between attribute groups.
- D3DXMESHOPT_DEVICEINDEPENDENT
- Affects the vertex cache size. Using this flag
specifies a default vertex cache size that works well on legacy hardware.
Remarks
The D3DXMESHOPT_STRIPREORDER and
D3DXMESHOPT_VERTEXCACHE optimization flags are mutually exclusive.
The D3DXMESHOPT_SHAREVB flag has been removed from this
enumeration. Use D3DXMESH_VB_SHARE instead, in D3DXMESH.
當一個mesh被使用D3DXMESHOPT_ATTRSORT參數(shù)來優(yōu)化后,mesh的幾何信息將按照屬性進行排序,這樣各個子集的頂點/索引將組成連續(xù)的塊(如圖10.3)。
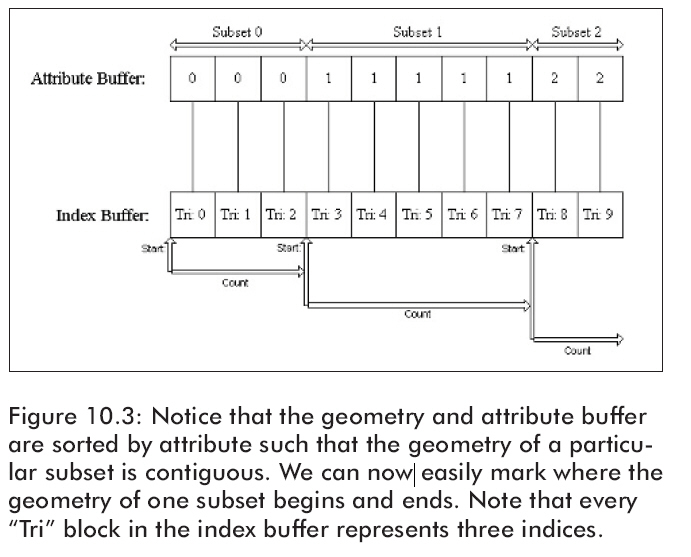
除了進行幾何信息的排序外,D3DXMESHOPT_ATTRSORT優(yōu)化項還將創(chuàng)建一個屬性表。該表是D3DXATTRIBUTERANGE結構的一個數(shù)組。在屬性表中的每一項對應mesh的一個子集并指示頂點/索引緩存中的一個連續(xù)連續(xù)內存塊,這個子集的幾何信息就包含在這個塊中。D3DXATTRIBUTERANGE結構的定義如下:
typedef
struct _D3DXATTRIBUTERANGE {
DWORD AttribId;
DWORD FaceStart;
DWORD FaceCount;
DWORD VertexStart;
DWORD VertexCount;
} D3DXATTRIBUTERANGE;
|
AttribId
— 子集的ID。
FaceStart
— 該子集的面的起始值,F(xiàn)aceStart*3就是起始三角形在索引緩存中的序號。
FaceCount
— 在子集中的面(三角形)數(shù)。
VertexStart
— 該子集的起始頂點在頂點緩存中的序號。
VertexCount
— 在子集中的頂點數(shù)。
建立了屬性表以后,渲染一個子集就很容易了。僅僅查一下屬性表就能找出自己的幾何信息。注意如果沒有屬性表,每渲染一個子集就需要對屬性緩存進行一次線性搜索來找出子集包含的幾何信息。
可以使用下面的方法來訪問mesh的屬性表:
Retrieves either an attribute table for a mesh, or the
number of entries stored in an attribute table for a mesh.
HRESULT GetAttributeTable(
D3DXATTRIBUTERANGE * pAttribTable,
DWORD * pAttribTableSize
);
Parameters
- pAttribTable
- [in, out] Pointer to an array of
D3DXATTRIBUTERANGE structures, representing the entries in the mesh's
attribute table. Specify NULL to retrieve the value for pAttribTableSize.
- pAttribTableSize
- [in, out] Pointer to either the number of entries
stored in pAttribTable or a value to be filled in with the number of entries
stored in the attribute table for the mesh.
Return Values
If the method succeeds, the return value is D3D_OK. If
the method fails, the return value can be D3DERR_INVALIDCALL.
Remarks
An attribute table is created by ID3DXMesh::Optimize
and passing D3DXMESHOPT_ATTRSORT for the Flags parameter.
An attribute table is used to identify areas of the
mesh that need to be drawn with different textures, render states, materials,
and so on. In addition, the application can use the attribute table to hide
portions of a mesh by not drawing a given attribute identifier when drawing the
frame.
這個方法能夠做兩件事情:它可以返回屬性表的屬性數(shù),也可以用屬性數(shù)據(jù)來填充一個D3DXATTRIBUTERANGE結構數(shù)組。
要得到屬性表的元素個數(shù),可以就將第一個參數(shù)設置為0:
DWORD numSubsets = 0;
Mesh->GetAttributeTable(0,
&numSubsets);
|
一旦我們知道了屬性表的元素個數(shù),我們就能夠通過寫屬性表來填充一個D3DXATTRIBUTERANGE結構數(shù)組:
D3DXATTRIBUTERANGE table =
new D3DXATTRIBUTERANGE [numSubsets];
Mesh->GetAttributeTable(
table, &numSubsets );
|
我們能夠使用ID3DXMesh::SetAttributeTable方法來直接設置屬性表。
Sets the attribute table for a mesh and the number of
entries stored in the table.
HRESULT SetAttributeTable(
CONST D3DXATTRIBUTERANGE * pAttribTable,
DWORD cAttribTableSize
);
Parameters
- pAttribTable
- [in] Pointer to an array of D3DXATTRIBUTERANGE
structures, representing the entries in the mesh attribute table.
- cAttribTableSize
- [in] Number of attributes in the mesh attribute
table.
Return Values
If the method succeeds, the return value is D3D_OK. If
the method fails, the return value can be one of the following:
D3DERR_INVALIDCALL, E_OUTOFMEMORY.
Remarks
If an application keeps track of the information in an
attribute table, and rearranges the table as a result of changes to attributes
or faces, this method allows the application to update the attribute tables
instead of calling ID3DXMesh::Optimize again.
下面的代碼就是設置一個有12個子集的屬性表:
D3DXATTRIBUTERANGE
attributeTable[12];
// ...fill
attributeTable array with data
Mesh->SetAttributeTable(
attributeTable, 12);
|
對于mesh的某些操作,如優(yōu)化,有必要了解的是三角形之間的鄰接信息。Mesh的鄰接數(shù)組存儲了這些信息。
鄰接數(shù)組是一個DWORD數(shù)組,其中的每一項對應了mesh中的一個三角形。例如,第i項對應的三角形由以下三個索引值定義:
A
=
i
x3
B
=
i
x3 + 1
C
=
i
x3 + 2
注意,使用ULONG_MAX
= 4294967295表示該邊沒有鄰接三角形。我們也可以用-1來表示,因為-1轉換成DWORD就是ULONG_MAX。回想一下,DWORD就是一個unsigned32-bit整數(shù)。
因為每個三角形都有三條邊,所以它最多有三個鄰接三角形(如圖10.4)。
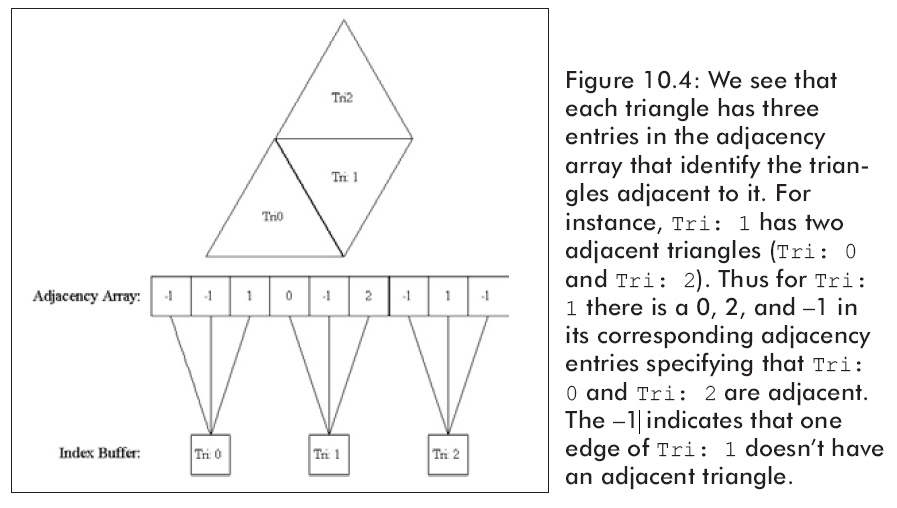
因此,鄰接數(shù)組必須有三項(ID3DXBaseMesh::GetNumFaces()*3)——
在mesh中每個三角形都可能有三個鄰接三角形。
很多D3Dxmesh創(chuàng)造函數(shù)都能輸出鄰接信息,但我們也可以使用下面的方法:
HRESULT
ID3DXMesh::GenerateAdjacency(
FLOAT fEpsilon,
DWORD* pAdjacency
);
|
fEpsilon
— 指示當兩個點距離有多近時,可以認為是一個點。當兩點間的距離小于epsilon時,可認為它們是同一個點。
pAdjacency
— 一個指向填充了鄰接信息的DWORD數(shù)組指針。
例子:
DWORD
adjacencyInfo[Mesh->GetNumFaces() * 3];
Mesh->GenerateAdjacency(0.001f, adjacencyInfo);
|