3.1頂點/索引緩存
頂點和索引緩存有相似的接口并且共享相似的方法;因此我們把它們合在一起講解。一個頂點緩存是一塊連續的存儲了頂點數據的內存。同樣的,一個索引緩存是一塊連續的存儲了索引數據的內存。我們使用頂點和索引緩存保存我們的數據是因為它們能被放置在顯存中。渲染顯存中的數據要比渲染系統內存中的數據快的多。
在代碼中,一個頂點緩存是通過IDirect3DVertexBuffer9接口來定義的。類似的,一個索引緩存是通過IDirect3DIndexBuffer9接口來定義。
3.1.1創建一個頂點和索引緩存
我們能使用下面兩個方法創建一個頂點緩存和索引緩存:
HRESULT
IDirect3DDevice9::CreateVertexBuffer(
UINT Length,
DWORD Usage,
DWORD FVF,
D3DPOOL Pool
IDirect3DVertexBuffer9** ppVertexBuffer,
HANDLE*
pSharedHandle
);
HRESULT
IDirect3DDevice9::CreateIndexBuffer(
UINT Length,
DWORD Usage,
D3DFORMAT Format,
D3DPOOL Pool,
IDirect3DIndexBuffer9** ppIndexBuffer,
HANDLE*
pSharedHandle
);
|
這兩個方法大部分參數是相同的,因此我們一起介紹它們。
Length ——
分配給緩存的字節大小。假如想得到一個能存儲8個頂點的頂點緩存,那么我們就要在頂點結構中設置這個參數為
8 * sizeof ( Vertex ) 。
Usage
—— 指定關于怎樣使用緩存的額外信息。這個值可以是0,沒有標記,或者是下面標記的一個或多個的組合:
D3DUSAGE_DYNAMIC——設置這個參數可以使緩存是動態的。
D3DUSAGE_POINTS——這個參數指定緩存存儲原始點。這個參數僅僅用在頂點緩沖中。
D3DUSAGE_SOFTWAREPROCESSING——使用軟件頂點處理
D3DUSAGE_WRITEONLY——指定應用程序只能寫緩存。它允許驅動程序分配最適合的內存地址作為寫緩存。注意如果從創建好的這種緩存中讀數據,將會返回錯誤信息。
FVF ——
存儲在緩存中的頂點格式
Pool
—— 緩存放置在哪一個內存池中
ppVertexBuffer
——返回創建好的頂點緩存的指針。
pSharedHandle
——沒有使用;設置為0。
Format
——指定索引的大小;使用D3DFMT_INDEX16設置16位索引,使用D3DFMT_INDEX32設置32位索引。注意并非所有設備都支持32位索引;請檢查設備能力。
ppIndexBuffer
——返回創建好的索引緩存的指針。
注意:不使用D3DUSAGE_DYNAMIC參數創建的緩存被叫做靜態緩存。靜態緩存通常被放置在顯存中,在其中的數據能被很有效的處理。然而,對于靜態緩存,從中讀取和寫入數據是很慢的,因為訪問顯存是很慢的。因為這個原因我們用靜態緩存存儲靜態數據(不需要被經常改變的數據)。地形和建筑物是很好的候選例子,因為在應用程序中他們通常不需要被改變。靜態緩存應該在應用程序初始化的時候就被填充好,而不是在運行時才做。
注意:使用D3DUSAGE_DYNAMIC參數創建的緩存被叫做動態緩存。動態緩存通常被放在AGP內存中,這種內存中的數據能被很快的更新。處理動態緩存中的數據不會比處理靜態緩存中的數據快,因為這些數據必須在渲染前被轉移到顯存中,動態緩存的好處是它們能夠被稍微快點地被更新(比CPU寫快)。因此,假如你需要經常更新緩存中的數據,那么你就應該使用動態緩存。粒子系統是很好的一個應用,因為它們是動態的,并且他們通常每一幀都會被更新。
注意:在程序中讀取顯存和AGP內存都是非常慢的。因此,假如你在運行時需要讀取你的幾何物體,最好的方案是指定一塊系統內存,往其中拷貝并且讀取數據。
下邊是創建一個靜態頂點緩存的例子,該緩存能存儲8個頂點。
IDirect3DVertexBuffer9* vb;
device->CreateVertexBuffer(
8 * sizeof( Vertex ), 0,
D3DFVF_XYZ, D3DPOOL_MANAGED, &vb, 0);
|
3.1.2
訪問緩沖內存
為了訪問一個頂點/索引緩存,我們需要得到一個指針。我們通過一個指針獲得緩存數據必須使用Lock方法。當我們訪問完緩存后必須對它解鎖。一旦有一個指向內存的指針,我們就能對它進行讀寫。
HRESULT
IDirect3DVertexBuffer9::Lock(
UINT OffsetToLock,
UINT SizeToLock,
BYTE** ppbData,
DWORD Flags
);
HRESULT
IDirect3DIndexBuffer9::Lock(
UINT OffsetToLock,
UINT SizeToLock,
BYTE** ppbData,
DWORD Flags
);
|
這兩個方法的參數都是完全相同的。
OffsetToLock
—— 偏移量,以字節為單位,從緩存開始位置到鎖定開始位置的距離。如圖3.1。
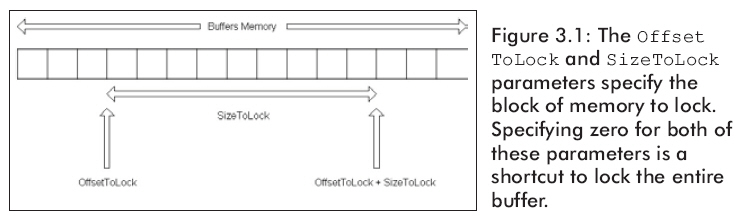
SizeToLock
——
鎖定的字節數。
ppbData
——
一個指向鎖定內存開始位置的指針。
Flags
—— 標記描述怎樣鎖定內存。它可能是0或者是下面參數中的1個或多個的組合:
D3DLOCK_DISCARD——這個參數僅僅會在動態緩存時被使用。它指示硬件丟棄緩存并返回一個指向新分配的緩存的指針。這是很有用,因為當我們存取一個新分配的緩存時它允許硬件繼續從丟棄的緩存渲染。這防止了硬件延遲。
D3DLOCK_NOOVERWRITE——這個參數僅僅會在動態緩存時被使用。它聲明你將向緩存中添加數據。即你不能向已經渲染的內存中寫數據。這是有好處的因為他允許你在添加新數據到緩存的同時讓硬件繼續渲染。
D3DLOCK_READONLY——這個參數聲明你鎖定的緩存只能從中讀取數據而不能寫數據。這允許一些內在的優化。
用參數D3DLOCK_DISCARD和D3DLOCK_NOOVERWRITE表明緩存的一部分被鎖定之后能繼續被使用。假如硬件配置允許這些標記被使用,則在對緩存進行鎖定時,其他的顯示操作就不會中斷。
下邊的例子展示了通常怎樣使用Lock方法。注意當我們使用完以后要調用Unlock方法。
Vertex* vertices;
_vb->Lock(0, 0, (void**)&vertices,
0); //
鎖定整個緩存
vertices[0] = Vertex(-1.0f,
0.0f, 2.0f); //
向緩存里寫頂點
vertices[1] = Vertex( 0.0f,
1.0f, 2.0f);
vertices[2] = Vertex( 1.0f,
0.0f, 2.0f);
_vb->Unlock();
//
當你訪問完緩存時,解鎖緩存
|
3.1.3
找回頂點和索引緩存信息
有時我們需要得到頂點/索引緩存信息。下面的例子示范了用于獲得這些信息的方法:
D3DVERTEXBUFFER_DESC
vbDescription;
_vertexBuffer->GetDesc(&vbDescription);
//
取得頂點緩存信息
D3DINDEXBUFFER_DESC
ibDescription;
_indexBuffer->GetDesc(&ibDescription);
//取得索引緩存信息
|
D3DVERTEXBUFFER_DESC和D3DINDEXBUFFER_DESC結構的定義如下:
Describes a vertex buffer.
typedef struct D3DVERTEXBUFFER_DESC {
D3DFORMAT Format;
D3DRESOURCETYPE Type;
DWORD Usage;
D3DPOOL Pool;
UINT Size;
DWORD FVF;
} D3DVERTEXBUFFER_DESC, *LPD3DVERTEXBUFFER_DESC;
Members
- Format
- Member of the D3DFORMAT enumerated type,
describing the surface format of the vertex buffer data.
- Type
- Member of the D3DRESOURCETYPE enumerated type,
identifying this resource as a vertex buffer.
- Usage
- Combination of one or more D3DUSAGE flags.
- Pool
- Member of the D3DPOOL enumerated type, specifying
the class of memory allocated for this vertex buffer.
- Size
- Size of the vertex buffer, in bytes.
- FVF
- Combination of D3DFVF that describes the vertex
format of the vertices in this buffer.
Defines resource types.
typedef enum D3DRESOURCETYPE
{
D3DRTYPE_SURFACE = 1,
D3DRTYPE_VOLUME = 2,
D3DRTYPE_TEXTURE = 3,
D3DRTYPE_VOLUMETEXTURE = 4,
D3DRTYPE_CubeTexture = 5,
D3DRTYPE_VERTEXBUFFER = 6,
D3DRTYPE_INDEXBUFFER = 7,
D3DRTYPE_FORCE_DWORD = 0x7fffffff,
} D3DRESOURCETYPE, *LPD3DRESOURCETYPE;
Constants
- D3DRTYPE_SURFACE
- Surface resource.
- D3DRTYPE_VOLUME
- Volume resource.
- D3DRTYPE_TEXTURE
- Texture resource.
- D3DRTYPE_VOLUMETEXTURE
- Volume texture resource.
- D3DRTYPE_CubeTexture
- Cube texture resource.
- D3DRTYPE_VERTEXBUFFER
- Vertex buffer resource.
- D3DRTYPE_INDEXBUFFER
- Index buffer resource.
- D3DRTYPE_FORCE_DWORD
- Forces this enumeration to compile to 32 bits in
size. Without this value, some compilers would allow this enumeration to
compile to a size other than 32 bits. This value is not used.
Describes an index buffer.
typedef struct D3DINDEXBUFFER_DESC {
D3DFORMAT Format;
D3DRESOURCETYPE Type;
DWORD Usage;
D3DPOOL Pool;
UINT Size;
} D3DINDEXBUFFER_DESC, *LPD3DINDEXBUFFER_DESC;
Members
- Format
- Member of the D3DFORMAT enumerated type,
describing the surface format of the index buffer data.
- Type
- Member of the D3DRESOURCETYPE enumerated type,
identifying this resource as an index buffer.
- Usage
- Combination of one or more of the following flags,
specifying the usage for this resource.
- D3DUSAGE_DONOTCLIP
- Set to indicate that the index buffer content
will never require clipping.
- D3DUSAGE_DYNAMIC
- Set to indicate that the index buffer requires
dynamic memory use. This is useful for drivers because it enables them
to decide where to place the buffer. In general, static index buffers
are placed in video memory and dynamic index buffers are placed in AGP
memory. Note that there is no separate static usage; if you do not
specify D3DUSAGE_DYNAMIC the index buffer is made static.
D3DUSAGE_DYNAMIC is strictly enforced through the D3DLOCK_DISCARD and
D3DLOCK_NOOVERWRITE locking flags. As a result, D3DLOCK_DISCARD and
D3DLOCK_NOOVERWRITE are only valid on index buffers created with
D3DUSAGE_DYNAMIC; they are not valid flags on static vertex buffers.
For more information about using dynamic index
buffers, see Using Dynamic Vertex and Index Buffers.
Note that D3DUSAGE_DYNAMIC cannot be specified
on managed index buffers. For more information, see Managing Resources
(Direct3D 9).
- D3DUSAGE_RTPATCHES
- Set to indicate when the index buffer is to be
used for drawing high-order primitives.
- D3DUSAGE_NPATCHES
- Set to indicate when the index buffer is to be
used for drawing N patches.
- D3DUSAGE_POINTS
- Set to indicate when the index buffer is to be
used for drawing point sprites or indexed point lists.
- D3DUSAGE_SOFTWAREPROCESSING
- Set to indicate that the buffer is to be used
with software processing.
- D3DUSAGE_WRITEONLY
- Informs the system that the application writes
only to the index buffer. Using this flag enables the driver to choose
the best memory location for efficient write operations and rendering.
Attempts to read from an index buffer that is created with this
capability can result in degraded performance.
- Pool
- Member of the D3DPOOL enumerated type, specifying
the class of memory allocated for this index buffer.
- Size
- Size of the index buffer, in bytes.