本篇是創建游戲內核(8)【C風格版】的續篇,關于該內核的細節說明請參考創建游戲內核(9)。
接口:
BOOL load_texture_from_file(LPDIRECT3DTEXTURE9* texture, pcstr filename, DWORD transparent, D3DFORMAT format);
BOOL create_texture(LPDIRECT3DTEXTURE9* texture, DWORD width, DWORD height, D3DFORMAT format);
BOOL clone_texture(LPDIRECT3DTEXTURE9* dest_texture, const LPDIRECT3DTEXTURE9 src_texture);
long get_texture_width(const LPDIRECT3DTEXTURE9 texture);
long get_texture_height(const LPDIRECT3DTEXTURE9 texture);
long get_texture_format(const LPDIRECT3DTEXTURE9 texture);
BOOL draw_texture(ID3DXSprite* sprite,
const LPDIRECT3DTEXTURE9 texture,
const RECT* texture_src_rect,
long dest_x, long dest_y,
float x_scale, float y_scale,
D3DCOLOR color);
實現:
//-------------------------------------------------------------------
// Create texture object from specified file, you can specify transparent
// value and pixel format of the texture.
//-------------------------------------------------------------------
BOOL load_texture_from_file(LPDIRECT3DTEXTURE9* texture, pcstr filename, DWORD transparent, D3DFORMAT format)
{
// check condition first
if(filename == NULL)
return FALSE;
// create a texture from file
if(FAILED(D3DXCreateTextureFromFileEx(g_d3d_device, filename, D3DX_DEFAULT, D3DX_DEFAULT,
D3DX_DEFAULT, 0, format, D3DPOOL_MANAGED, D3DX_FILTER_TRIANGLE, D3DX_FILTER_TRIANGLE,
transparent, NULL, NULL, texture)))
{
return FALSE;
}
return TRUE;
}
//-------------------------------------------------------------------
// Creates a texture resource.
//-------------------------------------------------------------------
BOOL create_texture(LPDIRECT3DTEXTURE9* texture, DWORD width, DWORD height, D3DFORMAT format)
{
if(FAILED(g_d3d_device->CreateTexture(width, height, 0, 0, format, D3DPOOL_MANAGED, texture, NULL)))
return FALSE;
return TRUE;
}
//-------------------------------------------------------------------
// Clone d3d texture object from an existing IDirect3DTexture9 object
// instance.
//-------------------------------------------------------------------
BOOL clone_texture(LPDIRECT3DTEXTURE9* dest_texture, const LPDIRECT3DTEXTURE9 src_texture)
{
if(src_texture == NULL)
return FALSE;
// copy texture over, from source to dest.
D3DSURFACE_DESC surface_desc;
if(FAILED(src_texture->GetLevelDesc(0, &surface_desc)))
return FALSE;
g_d3d_device->CreateTexture(surface_desc.Width, surface_desc.Height, 0, 0,
surface_desc.Format, D3DPOOL_MANAGED, dest_texture, NULL);
D3DLOCKED_RECT src_rect, dest_rect;
// locks a rectangle on a texture resource
src_texture->LockRect(0, &src_rect, NULL, D3DLOCK_READONLY);
(*dest_texture)->LockRect(0, &dest_rect, NULL, 0);
memcpy(dest_rect.pBits, src_rect.pBits, src_rect.Pitch * surface_desc.Height);
// unlocks a rectangle on a texture resource
src_texture->UnlockRect(0);
(*dest_texture)->UnlockRect(0);
return TRUE;
}
//-------------------------------------------------------------------
// Get width of the texture.
//-------------------------------------------------------------------
long get_texture_width(const LPDIRECT3DTEXTURE9 texture)
{
if(texture == NULL)
return 0;
D3DSURFACE_DESC surface_desc;
if(FAILED(texture->GetLevelDesc(0, &surface_desc)))
return 0;
return surface_desc.Width;
}
//-------------------------------------------------------------------
// Get height of the texture.
//-------------------------------------------------------------------
long get_texture_height(const LPDIRECT3DTEXTURE9 texture)
{
if(texture == NULL)
return 0;
D3DSURFACE_DESC surface_desc;
if(FAILED(texture->GetLevelDesc(0, &surface_desc)))
return 0;
return surface_desc.Height;
}
//-------------------------------------------------------------------
// Get texture storage format.
//-------------------------------------------------------------------
long get_texture_format(const LPDIRECT3DTEXTURE9 texture)
{
if(texture == NULL)
return D3DFMT_UNKNOWN;
D3DSURFACE_DESC surface_desc;
if(FAILED(texture->GetLevelDesc(0, &surface_desc)))
return D3DFMT_UNKNOWN;
return surface_desc.Format;
}
//-------------------------------------------------------------------
// Draw a 2D portion of texture to device.
//-------------------------------------------------------------------
BOOL draw_texture(ID3DXSprite* sprite,
const LPDIRECT3DTEXTURE9 texture,
const RECT* texture_src_rect,
long dest_x, long dest_y,
float x_scale, float y_scale,
D3DCOLOR color)
{
// check condition
if(texture == NULL || sprite == NULL)
return FALSE;
// set the portion of the source texture
D3DXMATRIX transform_matrix(x_scale, 0, 0, 0,
0, y_scale, 0, 0,
0, 0, 1, 0,
0, 0, 0, 1);
// sets the sprite transforma
sprite->SetTransform(&transform_matrix);
D3DXVECTOR3 dest_pos = D3DXVECTOR3((float)dest_x, (float)dest_y, 0);
// adds a sprite to the list of batched sprites
if(FAILED(sprite->Draw(texture, texture_src_rect, NULL, &dest_pos, color)))
return FALSE;
return TRUE;
}
測試代碼:
/***********************************************************************************
PURPOSE:
Test D3D texture function.
***********************************************************************************/
#include "core_common.h"
#include "core_framework.h"
#include "core_graphics.h"
#include "core_tool.h"
typedef struct DATA
{
ID3DXSprite* sprite;
IDirect3DTexture9* texture;
RECT texture_rect;
} *DATA_PTR;
//--------------------------------------------------------------------------------
// Initialize data for game.
//--------------------------------------------------------------------------------
BOOL game_init(void* data)
{
DATA_PTR in_data = (DATA_PTR) data;
// Create Direct3D and Direct3DDevice object
if(! create_display(g_hwnd, get_client_width(g_hwnd), get_client_height(g_hwnd), 16, TRUE, FALSE))
return FALSE;
// load the texture map
if(! load_texture_from_file(&in_data->texture, "tiger1.jpg", 0, D3DFMT_UNKNOWN))
return FALSE;
g_d3d_device->SetTexture(0, in_data->texture);
RECT& rect = in_data->texture_rect;
rect.left = 0;
rect.top = 0;
rect.right = get_window_width(g_hwnd);
rect.bottom = get_window_height(g_hwnd);
// create sprite
if(FAILED(D3DXCreateSprite(g_d3d_device, &in_data->sprite)))
return FALSE;
return TRUE;
}
//--------------------------------------------------------------------------------
// Render every game frame.
//--------------------------------------------------------------------------------
BOOL game_frame(void* data)
{
DATA_PTR in_data = (DATA_PTR) data;
clear_display_buffer(D3DCOLOR_RGBA(0, 0, 0, 255));
IDirect3DTexture9* texture = in_data->texture;
if(SUCCEEDED(g_d3d_device->BeginScene()))
{
ID3DXSprite* sprite = in_data->sprite;
if(SUCCEEDED(sprite->Begin(0)))
{
draw_texture(sprite, texture, &in_data->texture_rect, 0, 0, 1, 1, 0xFFFFFFFF);
sprite->End();
}
g_d3d_device->EndScene();
}
present_display();
return TRUE;
}
//--------------------------------------------------------------------------------
// Release all game resources.
//--------------------------------------------------------------------------------
BOOL game_shutdown(void* data)
{
DATA_PTR in_data = (DATA_PTR) data;
release_com(in_data->sprite);
release_com(in_data->texture);
destroy_display();
return TRUE;
}
//--------------------------------------------------------------------------------
// Main function, routine entry.
//--------------------------------------------------------------------------------
int WINAPI WinMain(HINSTANCE inst, HINSTANCE pre_inst, LPSTR cmd_line, int cmd_show)
{
DWORD client_width = 800;
DWORD client_height = 520;
DWORD x_pos = (get_screen_width() - client_width) / 2;
DWORD y_pos = (get_screen_height() - client_height) / 4;
if(! build_window(inst, "texture class", "texture test", WS_OVERLAPPEDWINDOW,
x_pos, y_pos, client_width, client_height))
{
return -1;
}
DATA data;
memset(&data, 0, sizeof(data));
run_game(game_init, game_frame, game_shutdown, &data);
return 0;
}
截圖:
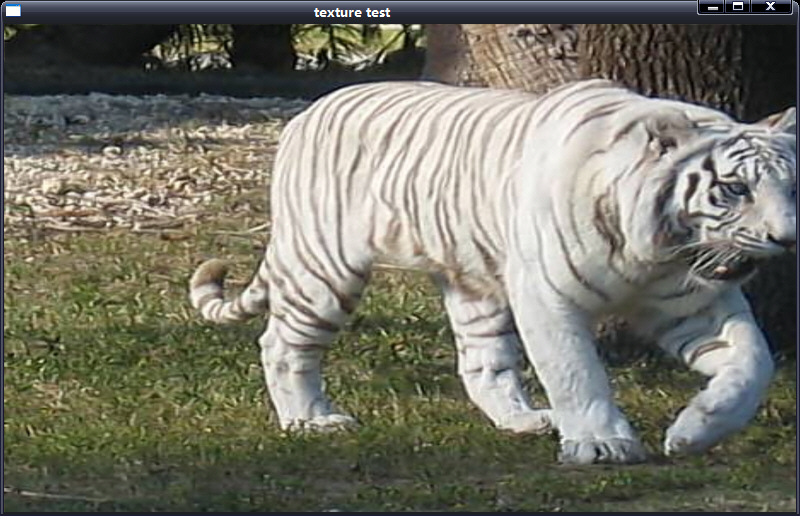