常用腳本實例:(以下都使用VBScript)
(主要講解腳本宿主和腳本運行時,腳本創建com和腳本引擎的擴展先不講,以后再講)
1
Creating and Controlling Files with FSO (Scripting.FileSystemObject)創建folder且collect 錯誤

' NewFolderEC.vbs
' Free example VBScript to create a folder with error-correcting Code.
' Author Guy Thomas http://computerperformance.co.uk/
' Version 2.6 - May 2005
' ---------------------------------------------------------------'

Option Explicit
Dim objFSO, objFolder, objShell, strDirectory
strDirectory = "c:\logs"

' Create the File System Object
Set objFSO = CreateObject("Scripting.FileSystemObject")

' Note If..Exists. Then, Else
End If construction
If objFSO.FolderExists(strDirectory) Then
Set objFolder = objFSO.GetFolder(strDirectory)
WScript.Echo strDirectory & " already created "
Else
Set objFolder = objFSO.CreateFolder(strDirectory)
WScript.Echo "Just created " & strDirectory
End If

If err.number = vbEmpty then
Set objShell = CreateObject("WScript.Shell")
objShell.run ("Explorer" &" " & strDirectory & "\" )
Else WScript.echo "VBScript Error: " & err.number
End If

WScript.Quit

' End of Sample VBScript to create a folder with error-correcting Code


創建文件且檢查文件是否已經存在

' NewFileEC.vbs
' Sample VBScript to create a file with error-correcting Code
' Author Guy Thomas http://computerperformance.co.uk/
' Version 1.6 - June 2005
' ---------------------------------------------------------------'

Option Explicit
Dim objFSO, objFolder, objShell, objFile
Dim strDirectory, strFile
strDirectory = "e:\logs"
strFile = "\Summer.txt"

' Create the File System Object
Set objFSO = CreateObject("Scripting.FileSystemObject")

' Check that the strDirectory folder exists
If objFSO.FolderExists(strDirectory) Then
Set objFolder = objFSO.GetFolder(strDirectory)
Else
Set objFolder = objFSO.CreateFolder(strDirectory)
WScript.Echo "Just created " & strDirectory
End If

If objFSO.FileExists(strDirectory & strFile) Then
Set objFolder = objFSO.GetFolder(strDirectory)
Else
Set objFile = objFSO.CreateTextFile(strDirectory & strFile)
Wscript.Echo "Just created " & strDirectory & strFile
End If

set objFolder = nothing
set objFile = nothing

If err.number = vbEmpty then
Set objShell = CreateObject("WScript.Shell")
objShell.run ("Explorer" & " " & strDirectory & "\" )
Else WScript.echo "VBScript Error: " & err.number
End If

WScript.Quit

' End of VBScript to create a file with error-correcting Code


文件的末尾增加內容

' NewTextEC.vbs
' Sample VBScript to write to a file. With added error-correcting
' Author Guy Thomas http://computerperformance.co.uk/
' Version 1.5 - August 2005
' ---------------------------------------------------------------'

Option Explicit
Dim objFSO, objFolder, objShell, objTextFile, objFile
Dim strDirectory, strFile, strText
strDirectory = "e:\logs3"
strFile = "\Summer.txt"
strText = "Book Another Holiday"

' Create the File System Object
Set objFSO = CreateObject("Scripting.FileSystemObject")

' Check that the strDirectory folder exists
If objFSO.FolderExists(strDirectory) Then
Set objFolder = objFSO.GetFolder(strDirectory)
Else
Set objFolder = objFSO.CreateFolder(strDirectory)
WScript.Echo "Just created " & strDirectory
End If

If objFSO.FileExists(strDirectory & strFile) Then
Set objFolder = objFSO.GetFolder(strDirectory)
Else
Set objFile = objFSO.CreateTextFile(strDirectory & strFile)
Wscript.Echo "Just created " & strDirectory & strFile
End If

set objFile = nothing
set objFolder = nothing
' OpenTextFile Method needs a Const value
' ForAppending = 8 ForReading = 1, ForWriting = 2
Const ForAppending = 8

Set objTextFile = objFSO.OpenTextFile _
(strDirectory & strFile, ForAppending, True)

' Writes strText every time you run this VBScript
objTextFile.WriteLine(strText)
objTextFile.Close

' Bonus or cosmetic section to launch explorer to check file
If err.number = vbEmpty then
Set objShell = CreateObject("WScript.Shell")
objShell.run ("Explorer" &" " & strDirectory & "\" )
Else WScript.echo "VBScript Error: " & err.number
End If

WScript.Quit

' End of VBScript to write to a file with error-correcting Code


寫log data到文件
' EventLogFSOvbs
' Example VBScript to interogate the Event Log and create a file
' Author Guy Thomas http://computerperformance.co.uk/
' Version 1.8 - June 2005
' ---------------------------------------------------------'
Option Explicit

Dim objFso, objFolder, objWMI, objItem, objShell, strEventLog
Dim strFile, strComputer, strFolder, strFileName, strPath
Dim intEvent, intNumberID, intRecordNum, colLoggedEvents

' --------------------------------------------------------
' Set the folder and file name
' Set numbers
intNumberID = 17 ' Event ID Number
intRecordNum = 0

strComputer = "."
strFileName = "\Event" & intNumberID & ".txt"
strFolder = "e:\logs\eventlog"
strPath = strFolder & strFileName
strEventLog = "'System' "

' -----------------------------------------------------
' Section to create folder and hold file.
Set objFso = CreateObject("Scripting.FileSystemObject")
If objFSO.FolderExists(strFolder) Then
Set objFolder = objFSO.GetFolder(strFolder)
Else
Set objFolder = objFSO.CreateFolder(strFolder)
Wscript.Echo "Folder created " & strFolder
End If

Wscript.Echo " Press OK and Wait 30 seconds (ish)"
Set strFile = objFso.CreateTextFile(strPath, True)
Set objWMI = GetObject("winmgmts:" _
& "{impersonationLevel=impersonate}!\\" _
& strComputer & "\root\cimv2")
Set colLoggedEvents = objWMI.ExecQuery _
("Select * from Win32_NTLogEvent Where Logfile = " & strEventLog)

' -----------------------------------------
' Next section loops through ID properties

For Each objItem in colLoggedEvents
If objItem.EventCode = intNumberID Then

' Second Loop to filter only if they tried Administrator
strFile.WriteLine("Category: " & objItem.Category _
& " string " & objItem.CategoryString)
strFile.WriteLine("ComputerName: " & objItem.ComputerName)
strFile.WriteLine("Logfile: " & objItem.Logfile _
& " source " & objItem.SourceName)
strFile.WriteLine("EventCode: " & objItem.EventCode)
strFile.WriteLine("EventType: " & objItem.EventType)
strFile.WriteLine("Type: " & objItem.Type)
strFile.WriteLine("User: " & objItem.User)
strFile.WriteLine("Message: " & objItem.Message)
strFile.WriteLine (" ")
intRecordNum = intRecordNum +1
End If
Next

' Confirms the script has completed and opens the file
Set objShell = CreateObject("WScript.Shell")
objShell.run ("Explorer" &" " & strPath & "\" )

WScript.Quit

' End of Guy's Sample FSO VBScript

拷貝文件
' VBScript.
Dim FSO
Set FSO = CreateObject("Scripting.FileSystemObject")
FSO.CopyFile "c:\COMPlusLog.txt", "c:\x\"
拷貝文件夾
' VBScript.
Dim FSO
Set FSO = CreateObject("Scripting.FileSystemObject")
FSO.CopyFolder "c:\x", "c:\y"
2 讀寫注冊表
' VBScript.
Set Sh = CreateObject("WScript.Shell")
key = "HKEY_CURRENT_USER\"
Sh.RegWrite key & "WSHTest\", "testkeydefault"
Sh.RegWrite key & "WSHTest\string1", "testkeystring1"
Sh.RegWrite key & "WSHTest\string2", "testkeystring2", "REG_SZ"
Sh.RegWrite key & "WSHTest\string3", "testkeystring3", "REG_EXPAND_SZ"
Sh.RegWrite key & "WSHTest\int", 123, "REG_DWORD"
WScript.Echo Sh.RegRead(key & "WSHTest\")
WScript.Echo Sh.RegRead(key & "WSHTest\string1")
WScript.Echo Sh.RegRead(key & "WSHTest\string2")
WScript.Echo Sh.RegRead(key & "WSHTest\string3")
WScript.Echo Sh.RegRead(key & "WSHTest\int")
Sh.RegDelete key & "WSHTest\"
3 創建快捷方式
VBScript.
Dim Shell, DesktopPath, URL
Set Shell = CreateObject("WScript.Shell")
DesktopPath = Shell.SpecialFolders("Desktop")
Set URL = Shell.CreateShortcut(DesktopPath & "\MSDN Scripting.URL")
URL.TargetPath = "HTTP://MSDN.Microsoft.com/scripting/"
URL.Save
' VBScript.
Set Shell = CreateObject("WScript.Shell")
DesktopPath = Shell.SpecialFolders("Desktop")
Set link = Shell.CreateShortcut(DesktopPath & "\test.lnk")
link.Arguments = "1 2 3"
link.Description = "test shortcut"
link.HotKey = "CTRL+ALT+SHIFT+X"
link.IconLocation = "app.exe,1"
link.TargetPath = "c:\blah\app.exe"
link.WindowStyle = 3
link.WorkingDirectory = "c:\blah"
link.Save
以下主要使用WMI
4 列舉系統進程
' Process.vbs
' Free Sample VBScript to discover which processes are running
' Author Guy Thomas http://computerperformance.co.uk/
' Version 1.4 - December 2005
' -------------------------------------------------------'
Option Explicit
Dim objWMIService, objProcess, colProcess
Dim strComputer, strList

strComputer = "."

Set objWMIService = GetObject("winmgmts:" _
& "{impersonationLevel=impersonate}!\\" _
& strComputer & "\root\cimv2")

Set colProcess = objWMIService.ExecQuery _
("Select * from Win32_Process")

For Each objProcess in colProcess
strList = strList & vbCr & _
objProcess.Name
Next

WSCript.Echo strList
WScript.Quit

' End of List Process Example VBScript


看看它的結果嗎:
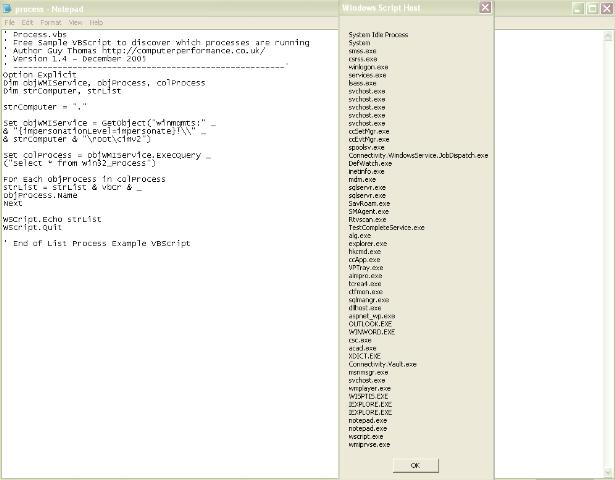
start stop 進程
' StartProcessLocal.vbs
' Free example VBScript to start a process (not interactive)
' Author Guy Thomas http://computerperformance.co.uk/
' Version 1.8 - December 2005
' -------------------------------------------------------'
Option Explicit
Dim objWMIService, objProcess, objCalc
Dim strShell, objProgram, strComputer, strExe

strComputer = "."
strExe = "Calc.exe"
' Connect to WMI
set objWMIService = getobject("winmgmts://"_
& strComputer & "/root/cimv2")

' Obtain the Win32_Process class of object.
Set objProcess = objWMIService.Get("Win32_Process")
Set objProgram = objProcess.Methods_( _
"Create").InParameters.SpawnInstance_
objProgram.CommandLine = strExe

'Execute the program now at the command line.
Set strShell = objWMIService.ExecMethod( _
"Win32_Process", "Create", objProgram)

WScript.echo "Created: " & strExe & " on " & strComputer
WSCript.Quit

' End of free example of a Process VBScript


' ProcessKillLocal.vbs
' Sample VBScript to kill a program
' Author Guy Thomas http://computerperformance.co.uk/
' Version 2.7 - December 2005
' ------------------------ -------------------------------'
Option Explicit
Dim objWMIService, objProcess, colProcess
Dim strComputer, strProcessKill
strComputer = "."
strProcessKill = "'calc.exe'"

Set objWMIService = GetObject("winmgmts:" _
& "{impersonationLevel=impersonate}!\\" _
& strComputer & "\root\cimv2")

Set colProcess = objWMIService.ExecQuery _
("Select * from Win32_Process Where Name = " & strProcessKill )
For Each objProcess in colProcess
objProcess.Terminate()
Next
WSCript.Echo "Just killed process " & strProcessKill _
& " on " & strComputer
WScript.Quit
' End of WMI Example of a Kill Process

物理和邏輯disks
' LogicalDisk.vbs
' Sample VBScript to interrogate a Logical disk with WMI
' Author Guy Thomas http://computerperformance.co.uk/
' Version 1.8 - November 2005
' -------------------------------------------------------------'
Option Explicit
Dim objWMIService, objItem, colItems, strComputer

On Error Resume Next
strComputer = "."

Set objWMIService = GetObject _
("winmgmts:\\" & strComputer & "\root\cimv2")
Set colItems = objWMIService.ExecQuery _
("Select * from Win32_LogicalDisk")

For Each objItem in colItems
Wscript.Echo "Computer: " & objItem.SystemName & VbCr & _
" ==================================" & VbCr & _
"Drive Letter: " & objItem.Name & vbCr & _
"Description: " & objItem.Description & vbCr & _
"Volume Name: " & objItem.VolumeName & vbCr & _
"Drive Type: " & objItem.DriveType & vbCr & _
"Media Type: " & objItem.MediaType & vbCr & _
"VolumeSerialNumber: " & objItem.VolumeSerialNumber & vbCr & _
"Size: " & Int(objItem.Size /1073741824) & " GB" & vbCr & _
"Free Space: " & Int(objItem.FreeSpace /1073741824) & _
" GB" & vbCr & _
"Quotas Disabled: " & objItem.QuotasDisabled & vbCr & _
"Supports DiskQuotas: " & objItem.SupportsDiskQuotas & vbCr & _
"Supports FileBasedCompression: " & _
objItem.SupportsFileBasedCompression & vbCr & _
"Compressed: " & objItem.Compressed & vbCr & _
""
Next

WSCript.Quit

' End of Sample DiskDrive VBScript

' DiskDriveLogical.vbs
' Sample VBScript to interrogate a physical disk with WMI
' Author Guy Thomas http://computerperformance.co.uk/
' Version 2.3 - November 2005
' --------------------------------------------------------------'
Option Explicit
Dim objWMIService, objItem, colItems, strComputer

' On Error Resume Next
strComputer = "."

Set objWMIService = GetObject("winmgmts:\\" _
& strComputer & "\root\cimv2")
Set colItems = objWMIService.ExecQuery(_
"Select * from Win32_DiskDrive")

For Each objItem in colItems
Wscript.Echo "Computer: " & objItem.SystemName & VbCr & _
"Status: " & objItem.Status & VbCr & _
" ==================================" & VbCr & _
"Name: " & objItem.Name & VbCr & _
"Description: " & objItem.Description & VbCr & _
"Signature: " & objItem.Signature & VbCr & _
"Manufacturer: " & objItem.Manufacturer & VbCr & _
"Model: " & objItem.Model & VbCr & _
"Size: " & Int(objItem.Size /(1073741824)) & " GB" & VbCr & _
"Number of Partitions: " & objItem.Partitions & VbCr & _
"Total Cylinders: " & objItem.TotalCylinders & VbCr & _
"Tracks PerCylinder: " & objItem.TracksPerCylinder & VbCr & _
"Total Heads: " & objItem.TotalHeads & VbCr & _
"Total Sectors: " & objItem.TotalSectors & VbCr & _
"Bytes PerSector: " & objItem.BytesPerSector & VbCr & _
"Sectors PerTrack: " & objItem.SectorsPerTrack & VbCr & _
"Total Tracks: " & objItem.TotalTracks & VbCr & _
" -------- SCSI Info ---------- "& VbCr & _
"SCSI TargetId: " & objItem.SCSITargetId & VbCr & _
"SCSI Bus: " & objItem.SCSIBus & VbCr & _
"SCSI Logical Unit: " & objItem.SCSILogicalUnit & VbCr & _
"SCSI Port: " & objItem.SCSIPort
Next
WSCript.Quit

' End of Sample DiskDrive VBScript
獲得memory
' Memory.vbs
' Sample VBScript to discover how much RAM in computer
' Author Guy Thomas http://computerperformance.co.uk/
' Version 1.3 - August 2005
' -------------------------------------------------------'
Option Explicit
Dim objWMIService, objComputer, colComputer
Dim strLogonUser, strComputer

strComputer = "."

Set objWMIService = GetObject("winmgmts:" _
& "{impersonationLevel=impersonate}!\\" _
& strComputer & "\root\cimv2")
Set colComputer = objWMIService.ExecQuery _
("Select * from Win32_ComputerSystem")

For Each objComputer in colComputer
Wscript.Echo "System Name: " & objComputer.Name _
& vbCr & "Total RAM " & objComputer.TotalPhysicalMemory
Next

WScript.Quit

' End of free example of Memory WMI / VBScript

打開百度:
Dim objIE
Set objIE = WScript.CreateObject ("InternetExplorer.Application")
objIE.AddressBar = true
objIE.Visible = true
objIE.Navigate("www.baidu.com")
刪除你制定的services:
strComputer = "."
Set objWMIService = GetObject("winmgmts:" _
& "{impersonationLevel=impersonate}!\\" & strComputer & "\root\cimv2")
Set colListOfServices = objWMIService.ExecQuery _
("Select * from Win32_Service Where Name = 'DbService'")
For Each objService in colListOfServices
objService.StopService()
objService.Delete()
Next
internet 和server active directory 還有創建com等沒有講哦!
主要參考:
MS的document
http://computerperformance.co.uk/