第一天,神說要有math,要計算法線,于是就有了Ogre::math,有了法線計算。

/**//** Calculate a face normal, including the w component which is the offset from the origin. */
static Vector4 calculateFaceNormal(const Vector3& v1, const Vector3& v2, const Vector3& v3);

/**//** Calculate a face normal, no w-information. */
static Vector3 calculateBasicFaceNormal(const Vector3& v1, const Vector3& v2, const Vector3& v3);

/**//** Calculate a face normal without normalize, including the w component which is the offset from the origin. */
static Vector4 calculateFaceNormalWithoutNormalize(const Vector3& v1, const Vector3& v2, const Vector3& v3);

/**//** Calculate a face normal without normalize, no w-information. */
static Vector3 calculateBasicFaceNormalWithoutNormalize(const Vector3& v1, const Vector3& v2, const Vector3& v3);
文如其名,四個上帝之子是同胞胎,只為了一個目的:得到法線,和一個附加長度。
//-----------------------------------------------------------------------
Vector4 Math::calculateFaceNormal(const Vector3& v1, const Vector3& v2, const Vector3& v3)

{
Vector3 normal = calculateBasicFaceNormal(v1, v2, v3);
// Now set up the w (distance of tri from origin
return Vector4(normal.x, normal.y, normal.z, -(normal.dotProduct(v1)));
}
//-----------------------------------------------------------------------
Vector3 Math::calculateBasicFaceNormal(const Vector3& v1, const Vector3& v2, const Vector3& v3)

{
Vector3 normal = (v2 - v1).crossProduct(v3 - v1);
normal.normalise();
return normal;
}
//-----------------------------------------------------------------------
Vector4 Math::calculateFaceNormalWithoutNormalize(const Vector3& v1, const Vector3& v2, const Vector3& v3)

{
Vector3 normal = calculateBasicFaceNormalWithoutNormalize(v1, v2, v3);
// Now set up the w (distance of tri from origin)
return Vector4(normal.x, normal.y, normal.z, -(normal.dotProduct(v1)));
}
//-----------------------------------------------------------------------
Vector3 Math::calculateBasicFaceNormalWithoutNormalize(const Vector3& v1, const Vector3& v2, const Vector3& v3)

{
Vector3 normal = (v2 - v1).crossProduct(v3 - v1);
return normal;
}
上帝是多么的英明,在你千辛萬苦google后卻還是無果的時候,卻發(fā)現(xiàn)圣書上古老的筆跡是最好的詮釋。
第二天,上帝走在水面上,說要有一個面,于是就有了平面。
Ogre::MeshManager::getSingleton().createPlane("floor", Ogre::ResourceGroupManager::DEFAULT_RESOURCE_GROUP_NAME,
Plane(Vector3::UNIT_Y, 0), 200, 200, 10, 10, true, 1, 10, 10, Vector3::UNIT_Z);

// create a floor entity, give it a material, and place it at the origin
Ogre::Entity* floor = mSceneMgr->createEntity("Floor", "floor");
floor->setMaterialName("Examples/Rockwall");
floor->setCastShadows(false);
mSceneMgr->getRootSceneNode()->attachObject(floor);
第三天,神說要有點、也要有叉。點點叉叉才像世界。
1).點積
維基:
http://en.wikipedia.org/wiki/Dot_product
點積沒有其他的幾何意義,除了表示2個向量的角度:
同時在ogre代碼中:
inline Real Vector3::dotProduct(const Vector3& vec) const

{
return x * vec.x + y * vec.y + z * vec.z;
}
這里也可以看到一個互動效果的點積圖形程序:
http://xahlee.org/SpecialPlaneCurves_dir/ggb/Vector_Dot_Product.html
也可以看到在其他領域點積還是有意義的,例如物理
2).叉積
維基:
http://en.wikipedia.org/wiki/Cross_product
叉積的結果正好是面的法線向量。這個向量的絕對值正好是向量包圍體的體積\面積。
叉積的結果表示:
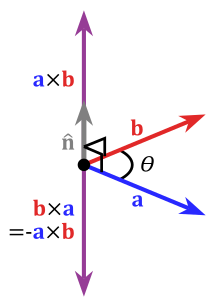
同時在ogre代碼中:
inline Vector3::Vector3 crossProduct( const Vector3& rkVector ) const

{
return Vector3(
y * rkVector.z - z * rkVector.y,
z * rkVector.x - x * rkVector.z

,
x * rkVector.y - y * rkVector.x);
}
3).四元數(shù)
一個四元數(shù)可以對應一個4*4矩陣,這是上帝的法則,作為它的子民,只有依其言行事。
這里你可以看到四元數(shù)---對應矩陣---模型的互動操作,I love It!當然,只板檣才可以讓演示程序跑起來,I 罰克中國恭饞褲襠。
http://www.euclideanspace.com/maths/geometry/rotations/conversions/quaternionToMatrix/program/index.htm
這個站還有四元數(shù)相關運算的詳細推導,例如四元數(shù)轉4*4矩陣。
4).矩陣
第四天,上帝想考驗世人的信仰,想看看每一顆心是不是紅的。
Entity *ent = NULL;
// mesh data to retrieve
size_t vertex_count;
size_t index_count;
Ogre::Vector3 *vertices;
unsigned long *indices;

// get the mesh information
GetMeshInformation(ent->getMesh(), vertex_count, vertices, index_count, indices,
ent->getParentNode()->_getDerivedPosition(),
ent->getParentNode()->_getDerivedOrientation(),
ent->getParentNode()->getScale());

// test for hitting individual triangles on the mesh
for (int i = 0; i < static_cast<int>(index_count); i += 3)


{
// check for a hit against this triangle
//std::pair<bool, Ogre::Real> hit = Ogre::Math::intersects(ray, vertices[indices[i]],
// vertices[indices[i+1]], vertices[indices[i+2]], true, false);
Vector3 noraml = Ogre::Math::calculateBasicFaceNormalWithoutNormalize (vertices[indices[i]], vertices[indices[i+1]], vertices[indices[i+2]] );
Vector3 center = (vertices[indices[i]] + vertices[indices[i+1]])/2;
center = (center+vertices[indices[i+2]] )/2;
mPoints.push_back(center);
mPoints.push_back(center + noraml );
}

// free the verticies and indicies memory
delete[] vertices;
delete[] indices;


/**////////////////////////////////////////////////////////////////////////
// Get the mesh information for the given mesh.
// Code found on this forum link: http://www.ogre3d.org/wiki/index.php/RetrieveVertexData
void DynLine::GetMeshInformation(const Ogre::MeshPtr mesh,
size_t &vertex_count,
Ogre::Vector3* &vertices,
size_t &index_count,
unsigned long* &indices,
const Ogre::Vector3 &position,
const Ogre::Quaternion &orient,
const Ogre::Vector3 &scale)
如此,上帝一一查明了世上的每一個生命,不管這個點式多么的渺小或微不足道,上帝均能一一檢視。這里,在每一個面上都計算出了法線向量。
第五天,修改了Node要記得update。
void Node::needUpdate(bool forceParentUpdate)