? WindowsAPI里的多線程簡單例子,先記下來,以后再學學
#include?<windows.h>
#include?<iostream>
using?namespace?std;
//線程處理函數
DWORD?WINAPI?Fun1(LPVOID?lpParam);
DWORD?WINAPI?Fun2(LPVOID?lpParam);
int?tickets?=?20;
void?main()
{
????HANDLE?hThread1,?hThread2;
????hThread1?=?CreateThread(NULL,?0,?Fun1,?NULL,?0,?NULL);
????hThread2?=?CreateThread(NULL,?0,?Fun2,?NULL,?0,?NULL);
????CloseHandle(hThread1);
????CloseHandle(hThread2);
????cin.get();
}
DWORD?WINAPI?Fun1(LPVOID?lpParam)
{
????while(true)
????{
????????if(tickets?>?0)
????????????cout<<"Thread1?sell?ticket?:?"<<tickets--<<endl;
????????else?break;
????}
????return?0;
}
DWORD?WINAPI?Fun2(LPVOID?lpParam)
{
????while(true)
????{
????????if(tickets?>?0)
????????????cout<<"Thread2?sell?ticket?:?"<<tickets--<<endl;
????????else?break;
????}
????return?0;
}
這里在main里用兩個函數Fun1和Fun2建了兩個線程,有一個全局變量ticket=20表示20張票,分由兩個線程進行出售。
但是這個程序運行起來結果會出現混亂和不確定:




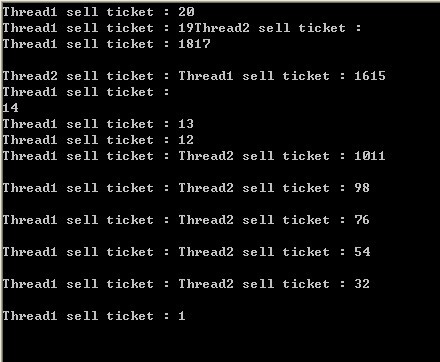
原因很簡單,在操作系統里都學過關于同步的問題,這里的兩個線程之間就沒有對臨界資源ticket進行同步。類似于操作系統里的PV操作,可以將程序改為:
#include?<windows.h>
#include?<iostream>
using?namespace?std;
//線程處理函數
DWORD?WINAPI?Fun1(LPVOID?lpParam);
DWORD?WINAPI?Fun2(LPVOID?lpParam);
int?tickets?=?20;
HANDLE?hMutex;
void?main()
{
????hMutex?=?CreateMutex(NULL,?false,?NULL);
????HANDLE?hThread1,?hThread2;
????hThread1?=?CreateThread(NULL,?0,?Fun1,?NULL,?0,?NULL);
????hThread2?=?CreateThread(NULL,?0,?Fun2,?NULL,?0,?NULL);
????CloseHandle(hThread1);
????CloseHandle(hThread2);
????cin.get();
}
DWORD?WINAPI?Fun1(LPVOID?lpParam)
{
????while(true)
????{
????????WaitForSingleObject(hMutex,?INFINITE);
????????if(tickets?>?0)
????????????cout<<"Thread1?sell?ticket?:?"<<tickets--<<endl;
????????else?break;
????????ReleaseMutex(hMutex);
????}
????return?0;
}
DWORD?WINAPI?Fun2(LPVOID?lpParam)
{
????while(true)
????{
????????WaitForSingleObject(hMutex,?INFINITE);
????????if(tickets?>?0)
????????????cout<<"Thread2?sell?ticket?:?"<<tickets--<<endl;
????????else?break;
????????ReleaseMutex(hMutex);
????}
????return?0;
}
這里用了一個信息量Mutex來對ticket進行同步操作,進程要操作ticket之前必須先獲得信號量,使用完之后釋放以使整個過程能繼續下去。
最后得到正常的結果為: