引擎的粒子系統我一直在考慮,以前也設計過,但是感覺以前做過的過于復雜,這里我本著讓粒子系統的接口盡可能的簡單,功能盡可能的強大,我把粒子系統設計成了這樣的形式:
1
2 typedef void(G_CALL *InitParticleFn)(Particle* p,float time);
3 typedef void(G_CALL *UpdateParticleFn)(Particle* p,float time);
4
5 ////////////////////////////////////////////////////////////
6 /// 定義引擎粒子系統描述符
7 ////////////////////////////////////////////////////////////
8 struct ParticleSystemDesc
9 {
10 ParticleSystemDesc():texture_id(0),
11 init_fn(NULL),
12 update_fn(NULL),
13 particle_number(2000),
14 life_span(8.0f),
15 birth_interval(life_span/(float)particle_number),
16 batch_particles(1),
17 particle_size(1.0f)
18 {
19 }
20 ~ParticleSystemDesc(){}
21
22 int texture_id;
23 InitParticleFn init_fn;
24 UpdateParticleFn update_fn;
25 int particle_number;
26 float life_span;
27 float birth_interval;
28 int batch_particles;
29 float particle_size;
30 };
31
32 ////////////////////////////////////////////////////////////
33 /// 定義引擎粒子系統類
34 ////////////////////////////////////////////////////////////
35 class ParticleSystem : NonCopyable, public Renderable
36 {
37 public:
38 ////////////////////////////////////////////////////////
39 /// 構造引擎粒子系統(傳入的描述符必須要有粒子初始化和更新回調函數)
40 ////////////////////////////////////////////////////////
41 ParticleSystem(const ParticleSystemDesc &desc):desc(desc){}
42 ~ParticleSystem(){}
43 public:
44 void BeginRender(){}
45 void AfterRender(){}
46
47 protected:
48 ParticleSystemDesc desc;
49 };
提供了2個粒子回調函數一個是粒子初始話的回調函數-表明初始化粒子的方式
一個是粒子更新回調函數-表明如何更新一個例子
通過粒子系統描述符來作為用戶和引擎的接口參數.
下面這是幾幅貼圖:
1.
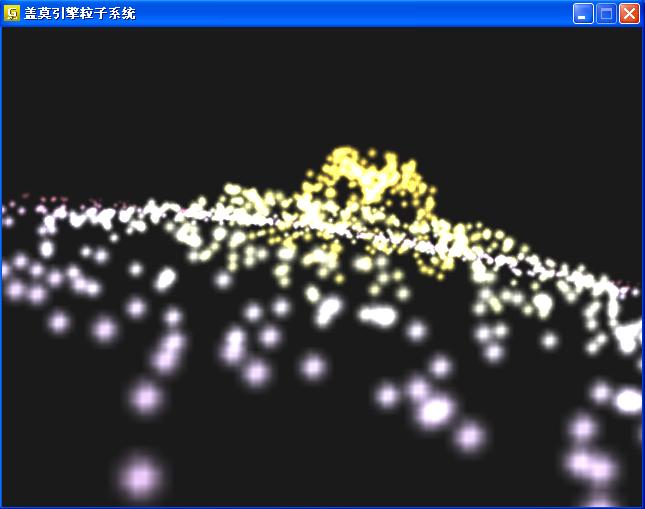
2.
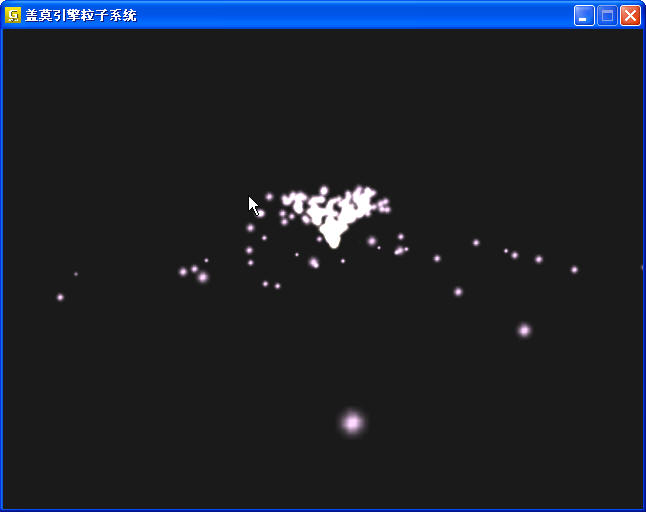
3.
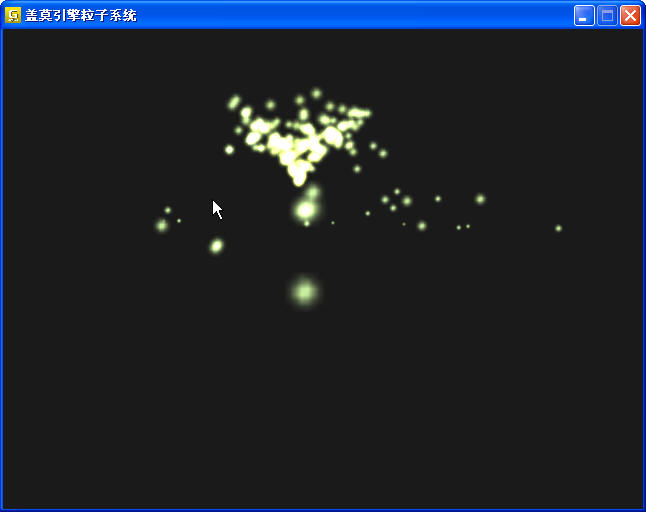
相關的代碼如下:
1 #include <GEngine/Main.hpp>
2
3 #define WIN_WIDTH 640
4 #define WIN_HEIGHT 480
5
6 //! 粒子紋理
7 static const unsigned char particle_texture[] =
8 {
9 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
10 0x00, 0x00, 0x11, 0x22, 0x22, 0x11, 0x00, 0x00,
11 0x00, 0x11, 0x33, 0x88, 0x77, 0x33, 0x11, 0x00,
12 0x00, 0x22, 0x88, 0xff, 0xee, 0x77, 0x22, 0x00,
13 0x00, 0x22, 0x77, 0xee, 0xff, 0x88, 0x22, 0x00,
14 0x00, 0x11, 0x33, 0x77, 0x88, 0x33, 0x11, 0x00,
15 0x00, 0x00, 0x11, 0x33, 0x22, 0x11, 0x00, 0x00,
16 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00
17 };
18
19 //! 粒子大小
20 #define PARTICLE_SIZE 0.7f
21
22 //! 粒子重力加速度
23 #define GRAVITY 9.8f
24
25 //! 粒子初始化速度
26 #define VELOCITY 8.0f
27
28 //! 粒子噴泉高度
29 #define FOUNTAIN_HEIGHT 3.0f
30
31 //! 粒子噴泉半徑
32 #define FOUNTAIN_RADIUS 1.6f
33
34 //! 粒子紋理大小
35 #define P_TEX_WIDTH 8
36 #define P_TEX_HEIGHT 8
37
38 //! 粒子彈性碰撞摩擦力系數(1為無摩擦,0為最大摩擦)
39 #define FRICTION 0.75f
40
41 #define FOUNTAIN_R2 (FOUNTAIN_RADIUS+PARTICLE_SIZE/2)*(FOUNTAIN_RADIUS+PARTICLE_SIZE/2)
42
43 ////////////////////////////////////////////////////////////
44 /// 給出一個初始化粒子的方法
45 ////////////////////////////////////////////////////////////
46 void G_CALL InitParticle(core::Particle* p,float t);
47
48 ////////////////////////////////////////////////////////////
49 /// 更新粒子函數
50 ////////////////////////////////////////////////////////////
51 void G_CALL UpdateParticle(core::Particle* p,float time);
52
53 ////////////////////////////////////////////////////////////
54 /// 場景旋轉和偏移
55 ////////////////////////////////////////////////////////////
56 float TransForm(double t);
57
58 ////////////////////////////////////////////////////////////
59 /// 構造紋理
60 ////////////////////////////////////////////////////////////
61 void BuildTexture(GLuint& texture_id);
62
63 ////////////////////////////////////////////////////////////
64 /// 渲染場景
65 ////////////////////////////////////////////////////////////
66 void RenderScene();
67
68 core::Device* device = NULL;
69 libmath::TriTable* table = NULL;
70
71 int main(int argc, char **argv)
72 {
73 int i;
74 double t0, t;
75
76 core::VideoMode mode;
77 mode.width = WIN_WIDTH;
78 mode.height = WIN_HEIGHT;
79
80 device = core::InitDevice("蓋莫引擎粒子系統",false,mode);
81 table = device->GetTriTable();
82
83 core::ParticleSystemDesc desc;
84
85 GLuint texture_id;
86 BuildTexture(texture_id);
87 desc.texture_id = texture_id;
88 desc.init_fn = &InitParticle;
89 desc.update_fn = &UpdateParticle;
90 desc.batch_particles = 80;
91 desc.particle_size = 0.6f;
92 desc.life_span = 8;
93
94 core::ParticleSystem* ps = device->GetParticleSystem(desc);
95
96 t0 = device->GetTime();
97 BEGIN_LOOP(device)
98 t = device->GetTime() - t0;
99 RenderScene();
100 float dt = TransForm(t);
101 ps->Render();
102 END_LOOP(device)
103
104 device->Close();
105 device->Drop();
106
107 return 0;
108 }
109
110 void BuildTexture(GLuint& texture_id)
111 {
112 glGenTextures( 1, &texture_id );
113 glBindTexture( GL_TEXTURE_2D, texture_id);
114 glPixelStorei( GL_UNPACK_ALIGNMENT, 1 );
115 glTexParameteri( GL_TEXTURE_2D, GL_TEXTURE_WRAP_S, GL_CLAMP );
116 glTexParameteri( GL_TEXTURE_2D, GL_TEXTURE_WRAP_T, GL_CLAMP );
117 glTexParameteri( GL_TEXTURE_2D, GL_TEXTURE_MAG_FILTER, GL_LINEAR );
118 glTexParameteri( GL_TEXTURE_2D, GL_TEXTURE_MIN_FILTER, GL_LINEAR );
119 glTexImage2D(GL_TEXTURE_2D, 0, GL_LUMINANCE, P_TEX_WIDTH, P_TEX_HEIGHT,
120 0, GL_LUMINANCE, GL_UNSIGNED_BYTE, particle_texture);
121 }
122
123 ////////////////////////////////////////////////////////////
124 /// 場景旋轉和偏移
125 ////////////////////////////////////////////////////////////
126 float TransForm(double t)
127 {
128 double xpos, ypos, zpos, angle_x, angle_y, angle_z;
129 static double t_old = 0.0;
130 float dt = (float)(t-t_old);
131 t_old = t;
132
133 angle_x = 90.0 - 10.0;
134 angle_y = 10.0 * sin( 0.3 * t );
135 angle_z = 10.0 * t;
136 glRotated( -angle_x, 1.0, 0.0, 0.0 );
137 glRotated( -angle_y, 0.0, 1.0, 0.0 );
138 glRotated( -angle_z, 0.0, 0.0, 1.0 );
139
140 xpos = 15.0 * sin( (M_PI/180.0) * angle_z ) +
141 2.0 * sin( (M_PI/180.0) * 3.1 * t );
142 ypos = -15.0 * cos( (M_PI/180.0) * angle_z ) +
143 2.0 * cos( (M_PI/180.0) * 2.9 * t );
144 zpos = 4.0 + 2.0 * cos( (M_PI/180.0) * 4.9 * t );
145 glTranslated( -xpos, -ypos, -zpos );
146 return dt;
147 }
148
149 void RenderScene()
150 {
151 glViewport( 0, 0, WIN_WIDTH,WIN_HEIGHT);
152 glClearColor( 0.1f, 0.1f, 0.1f, 1.0f );
153 glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT );
154 glMatrixMode( GL_PROJECTION );
155 glLoadIdentity();
156 gluPerspective(65.0, 640.0/480.0, 1.0, 60.0 );
157 glMatrixMode( GL_MODELVIEW );
158 glLoadIdentity();
159 }
160
161 ////////////////////////////////////////////////////////////
162 /// 給出一個初始化粒子的方法(t為粒子系統啟動時間(單位:秒))
163 ////////////////////////////////////////////////////////////
164 void G_CALL InitParticle(core::Particle* p,float t)
165 {
166 //! 初始化粒子位置
167 p->position = Vector3f(0,0,FOUNTAIN_HEIGHT);
168
169 //! 設定粒子初始z軸速度
170 p->velocity.z = 0.7f + (0.3/4096.0) * (float) (rand() & 4095);
171
172 //! 獲取隨機xy平面旋轉角度
173 float xy_angle = (2.0*M_PI/4096.0) * (float) (rand() & 4095);
174 p->velocity.x = 0.45f * table->CosTable(xy_angle);
175 p->velocity.y = 0.45f * table->SinTable(xy_angle);
176
177 //! 根據時間調整粒子初始速度
178 float velocity = VELOCITY*(0.8f + 0.1f*(float)(table->SinTable(0.5*t)+table->SinTable(0.31*t)));
179 p->velocity.x *= velocity;
180 p->velocity.y *= velocity;
181 p->velocity.z *= velocity;
182
183 //! 設定粒子初始顏色
184 p->color.red = 0.7f + 0.3f * table->SinTable(0.34*t + 0.1);
185 p->color.green = 0.6f + 0.4f * table->SinTable(0.63*t + 1.1);
186 p->color.blue = 0.6f + 0.4f * table->SinTable(0.91*t + 2.1);
187 }
188
189 ////////////////////////////////////////////////////////////
190 /// 更新粒子函數
191 ////////////////////////////////////////////////////////////
192 void G_CALL UpdateParticle(core::Particle* p,float time)
193 {
194 float dt = time;
195 //! 修正粒子生命
196 p->life = p->life - dt * (1.0f / 8.0f);
197 //! 修正粒子速度
198 p->velocity.z = p->velocity.z - GRAVITY *dt;
199 p->position += p->velocity * dt;
200
201 //! 處理簡單的碰撞
202 if( p->velocity.z < 0.0f )
203 {
204 if((p->position.x*p->position.x + p->position.y*p->position.y) < FOUNTAIN_R2 &&
205 p->position.z < (FOUNTAIN_HEIGHT + PARTICLE_SIZE/2) )
206 {
207 p->velocity.z = -FRICTION * p->velocity.z;
208 p->position.z = FOUNTAIN_HEIGHT + PARTICLE_SIZE/2 +
209 FRICTION * (FOUNTAIN_HEIGHT +
210 PARTICLE_SIZE/2 - p->position.z);
211 }
212
213 //! 當粒子碰撞到地面應該跳起來
214 else if( p->position.z < PARTICLE_SIZE/2 )
215 {
216 p->velocity.z = -FRICTION * p->velocity.z;
217 p->position.z = PARTICLE_SIZE/2 + FRICTION * (PARTICLE_SIZE/2 - p->position.z);
218 }
219 }
220 }
221
222
這個場景例子的原型源于glfw庫附帶粒子