90分鐘寫好的一個基于libgdx的android應用,有興趣的
下載看看,也算這段時間研究libgdx的總結吧。。。版本需要2.1及其以上版本,由于cppblog.com附件后綴上傳限制,下載
Star-Android.apk.7z后,請去掉.7z后綴,再安裝,程序名稱為--star。別忘記android手機的觸屏功能哦。。。
Mac下不知道怎么截屏,明天再上圖吧~
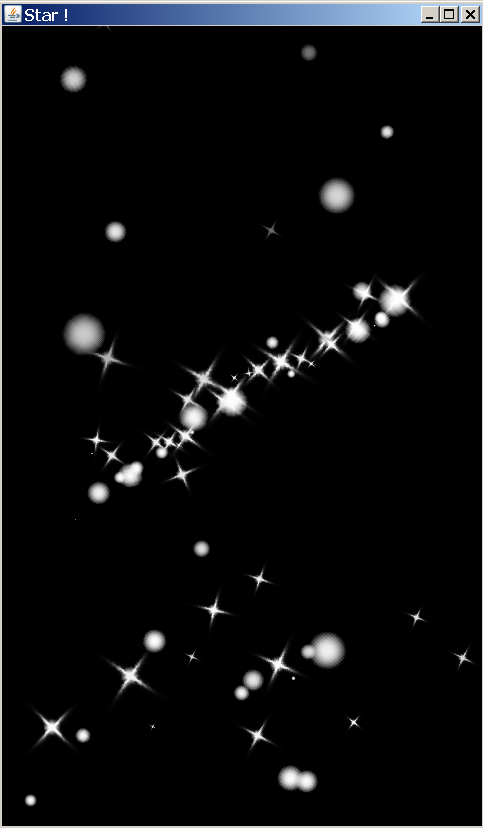
Source Code:
1 /**
2 * file: StarGame.java
3 * author: codejie (codejie@gmail.com)
4 * date: Jun 2, 2011 11:32:00 PM
5 */
6 package com.jie.android.gdx.star;
7
8 import com.badlogic.gdx.Game;
9 import com.badlogic.gdx.Gdx;
10 import com.badlogic.gdx.InputProcessor;
11 import com.badlogic.gdx.graphics.GL10;
12 import com.badlogic.gdx.graphics.Texture;
13 import com.badlogic.gdx.math.MathUtils;
14 import com.badlogic.gdx.scenes.scene2d.Action;
15 import com.badlogic.gdx.scenes.scene2d.OnActionCompleted;
16 import com.badlogic.gdx.scenes.scene2d.Stage;
17 import com.badlogic.gdx.scenes.scene2d.actions.FadeTo;
18 import com.badlogic.gdx.scenes.scene2d.actions.MoveTo;
19 import com.badlogic.gdx.scenes.scene2d.actions.Parallel;
20 import com.badlogic.gdx.scenes.scene2d.actions.RotateTo;
21 import com.badlogic.gdx.scenes.scene2d.actions.ScaleTo;
22 import com.badlogic.gdx.scenes.scene2d.actors.Image;
23
24 public class StarGame extends Game implements InputProcessor {
25
26 /* (non-Javadoc)
27 * @see com.badlogic.gdx.ApplicationListener#create()
28 */
29
30 private Stage stage = null;
31 private Texture ballTexture = null;
32 private Texture starTexture = null;
33
34 @Override
35 public void create() {
36 // TODO Auto-generated method stub
37 stage = new Stage(480, 800, true);
38
39 ballTexture = new Texture(Gdx.files.internal("data/ball.png"));
40 starTexture = new Texture(Gdx.files.internal("data/star.png"));
41
42 Gdx.input.setInputProcessor(this);
43 }
44
45 private void makeStar(boolean star, int x, int y, int size) {
46 Image img = null;
47
48 if (star == true) {
49 img = new Image("star", starTexture);
50 }
51 else {
52 img = new Image("ball", ballTexture);
53 if(size > 24)
54 size -= 24;
55 }
56 img.x = x;
57 img.y = y;
58 img.width = size;
59 img.height = size;
60
61 this.addAction(img);
62
63 stage.addActor(img);
64 }
65
66 private void addAction(final Image img) {
67
68 int duration = MathUtils.random(3, 60);
69 MoveTo moveto = MoveTo.$(img.x, 800, duration);
70 moveto.setCompletionListener(new OnActionCompleted() {
71 public void completed(Action action) {
72 stage.removeActor(img);
73 }
74 });
75
76 int rotate = MathUtils.random(360);
77 float scale = MathUtils.random(0.5f, 2.0f);
78 float fade = MathUtils.random(1.0f);
79 Action action = Parallel.$(
80 moveto,
81 ScaleTo.$(scale, scale, duration),
82 RotateTo.$(rotate, duration),
83 FadeTo.$(fade, duration)
84 );
85
86 img.action(action);
87 }
88
89 public void render() {
90 Gdx.gl.glClearColor(0,0,0,0);
91 Gdx.graphics.getGL10().glClear(GL10.GL_COLOR_BUFFER_BIT);
92
93 float delta = Gdx.graphics.getDeltaTime();
94
95 int roll = (int)(delta * 1000000);
96 if (roll % 15 == 0) {
97 makeStar(MathUtils.randomBoolean(), MathUtils.random(0, 480), MathUtils.random(0, 64), MathUtils.random(10, 64));
98 }
99
100 stage.act(delta);
101 stage.draw();
102 }
103
104 public void dispose() {
105 stage.dispose();
106 ballTexture.dispose();
107 starTexture.dispose();
108 }
109
110
111 /* (non-Javadoc)
112 * @see com.badlogic.gdx.InputProcessor#keyDown(int)
113 */
114 @Override
115 public boolean keyDown(int arg0) {
116 // TODO Auto-generated method stub
117 return false;
118 }
119
120 /* (non-Javadoc)
121 * @see com.badlogic.gdx.InputProcessor#keyTyped(char)
122 */
123 @Override
124 public boolean keyTyped(char arg0) {
125 // TODO Auto-generated method stub
126 return false;
127 }
128
129 /* (non-Javadoc)
130 * @see com.badlogic.gdx.InputProcessor#keyUp(int)
131 */
132 @Override
133 public boolean keyUp(int arg0) {
134 // TODO Auto-generated method stub
135 return false;
136 }
137
138 /* (non-Javadoc)
139 * @see com.badlogic.gdx.InputProcessor#scrolled(int)
140 */
141 @Override
142 public boolean scrolled(int arg0) {
143 // TODO Auto-generated method stub
144 return false;
145 }
146
147 /* (non-Javadoc)
148 * @see com.badlogic.gdx.InputProcessor#touchDown(int, int, int, int)
149 */
150 @Override
151 public boolean touchDown(int arg0, int arg1, int arg2, int arg3) {
152 // TODO Auto-generated method stub
153 return false;
154 }
155
156 /* (non-Javadoc)
157 * @see com.badlogic.gdx.InputProcessor#touchDragged(int, int, int)
158 */
159 @Override
160 public boolean touchDragged(int arg0, int arg1, int arg2) {
161 // TODO Auto-generated method stub
162
163 makeStar(MathUtils.randomBoolean(), arg0, 800 - arg1, MathUtils.random(10, 64));
164
165 return false;
166 }
167
168 /* (non-Javadoc)
169 * @see com.badlogic.gdx.InputProcessor#touchMoved(int, int)
170 */
171 @Override
172 public boolean touchMoved(int arg0, int arg1) {
173 // TODO Auto-generated method stub
174 return false;
175 }
176
177 /* (non-Javadoc)
178 * @see com.badlogic.gdx.InputProcessor#touchUp(int, int, int, int)
179 */
180 @Override
181 public boolean touchUp(int arg0, int arg1, int arg2, int arg3) {
182 // TODO Auto-generated method stub
183 return false;
184 }
185
186 }
187