所謂的單件類就是保證一個類僅有一個實例,并提供一個訪問它的全局訪問點。
Singleton可以看作是一種經過改進的全局變量,既在一個進程中只能有唯一的實例,不允許產生第二個這樣的對象。
雖然單件類是最簡單的設計模式,但仍需小心使用,主要需注意:
1.構造函數
既然是只能有一個實例,那么構造函數自然不能被外部隨意調用,所以需要將其聲明為私有(private),包括默認構造、拷貝構造及賦值操作。至于是否需要實現要看具體應用。實例的產生需要一個輔助的成員函數(類似getInstance或creatInstance)。
2.析構函數
需要定義全局唯一的變量,我們首先會想到的就是靜態(static),沒錯,單件類也是通過靜態成員指針變量來實現單一。我們往往習慣于在析構函數中對成員指針進行內存釋放,但在單件類中是不可以這樣操作的,因為delete會調用類的析構,所以在自己的析構中delete自己的對象就會造成遞歸析構(無窮盡的析構現象)。
UML類圖:
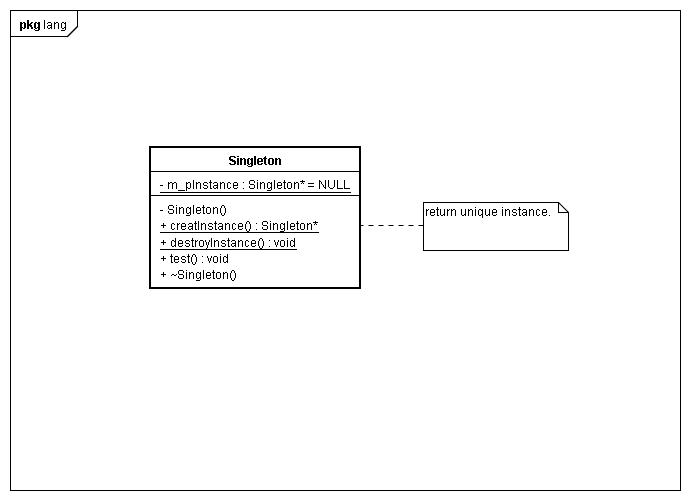
1)Singleton.hpp
1
/**//********************************************************************
2
* Copyright (c) 2010~2010 All Rights Resverved by wei.chen.
3
********************************************************************/
4
/**//**
5
* @file Singleton.hpp
6
* @brief Declare the class of Singleton.
7
* @version 0.1
8
* @since 0.1
9
* @author chenwei<76487974@qq.com>
10
* @date 2010-7-19 Created it
11
*/
12
13
#ifndef _SINGLETON_HPP
14
#define _SINGLETON_HPP
15
16
#include <iostream>
17
18
class Singleton
19

{
20
public:
21
~Singleton()
{
22
std::cout << "Singleton destructor." << std::endl;
23
}
24
25
static Singleton* creatInstance();
26
static void destroyInstance();
27
void test()
{
28
std::cout << "Singleton test." << std::endl;
29
}
30
31
private:
32
static Singleton* m_pInstance;
33
34
Singleton()
{
35
std::cout << "Singleton constructor." << std::endl;
36
}
37
38
Singleton(Singleton&);
39
Singleton& operator=(Singleton&);
40
};
41
42
#endif
43
44


2

3

4


5

6

7

8

9

10

11

12

13

14

15

16

17

18

19



20

21



22

23

24

25

26

27



28

29

30

31

32

33

34



35

36

37

38

39

40

41

42

43

44

2)Singleton.cpp
1
/**//********************************************************************
2
* Copyright (c) 2010~2010 All Rights Resverved by wei.chen.
3
********************************************************************/
4
/**//**
5
* @file Singleton.cpp
6
* @brief Implement the methods of the class Singleton.
7
* @version 0.1
8
* @since 0.1
9
* @author chenwei<76487974@qq.com>
10
* @date 2010-7-19 Created it
11
*/
12
13
#include "Singleton.hpp"
14
#include <stdlib.h>
15
16
Singleton* Singleton::m_pInstance = NULL;
17
18
/**//**
19
* @fn creatInstance
20
* @brief Create a Singleton instance.
21
* @return A pointer to Singleton Instance, or NULL if failed.
22
* @author wei.chen (2010-7-19)
23
*/
24
Singleton* Singleton::creatInstance()
25

{
26
std::cout << "Create the instance." << std::endl;
27
if (!m_pInstance)
{
28
m_pInstance = new Singleton();
29
if (!m_pInstance)
{
30
std::cout << "No memory to new for Singleton." << std::endl;
31
abort();
32
}
33
}
34
35
return m_pInstance;
36
}
37
38
/**//**
39
* @fn destroyInstance
40
* @brief Release the memory for destroying the instance.
41
* @author wei.chen (2010-7-19)
42
*/
43
void Singleton::destroyInstance()
44

{
45
std::cout << "Destroy the instance." << std::endl;
46
delete m_pInstance;
47
m_pInstance = NULL;
48
}
49


2

3

4


5

6

7

8

9

10

11

12

13

14

15

16

17

18


19

20

21

22

23

24

25



26

27



28

29



30

31

32

33

34

35

36

37

38


39

40

41

42

43

44



45

46

47

48

49

3)Main.cpp
1
/**//********************************************************************
2
* Copyright (c) 2010~2010 All Rights Resverved by wei.chen.
3
********************************************************************/
4
/**//**
5
* @file Main.cpp
6
* @brief The entrance of the program.
7
* @version 0.1
8
* @since 0.1
9
* @author chenwei<76487974@qq.com>
10
* @date 2010-7-19 Created it
11
*/
12
13
#include "Singleton.hpp"
14
15
/**//**
16
* @fn main
17
* @brief The entrance of the program.
18
* @return int
19
* @retval 0-normal
20
* @author wei.chen (2010-7-19)
21
*/
22
int main()
23

{
24
Singleton* singletonTest = Singleton::creatInstance();
25
if (!singletonTest)
{
26
std::cout << "Create Instance failed." << std::endl;
27
return -1;
28
}
29
30
singletonTest->test();
31
Singleton::destroyInstance();
32
33
return 0;
34
}
35


2

3

4


5

6

7

8

9

10

11

12

13

14

15


16

17

18

19

20

21

22

23



24

25



26

27

28

29

30

31

32

33

34

35
