Posted on 2010-05-02 20:18
Current 閱讀(2190)
評論(2) 編輯 收藏 引用 所屬分類:
Wild Magic 引擎解析
在維基百科中 hash 的解釋為:
散列函數(或散列算法,英語:Hash Function)是一種從任何一種數據中創建小的數字“指紋”的方法。該函數將數據打亂混合,重新創建一個叫做散列值的指紋。散列值通常用來代表一個短的隨機字母和數字組成的字符串。好的散列函數在輸入域中很少出現散列沖突。在散列表和數據處理中,不抑制沖突來區別數據,會使得數據庫記錄更難找到。
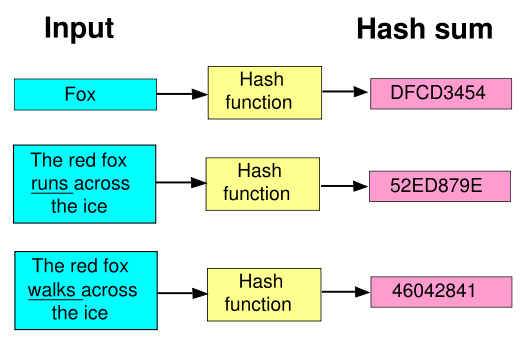
Wild Magic 中 Hash Table實現這里所指“指紋”是指:通過一個唯一的“量”(如:一個object的物理地址的指針值),在二叉樹中快速的查找到相對應的變量。
具體代碼:
THashTable.hpp
1
#ifndef _HASHTABLE_HPP_
2
#define _HASHTABLE_HPP_
3
4
#include "FoundationLIB.hpp"
5
6
#include "System.hpp"
7
8
/**//*
9
* 利用hash函數 實現對鏈表的訪問運算量達到和數組訪問相當的效率
10
*/
11
namespace PX2
12

{
13
/**//// 哈希表模板類
14
template<class TKEY, class TVALUE>
15
class THashTable
16
{
17
public:
18
THashTable ( int iTableSize );
19
~THashTable ();
20
21
int GetQuantity () const;
22
23
bool Insert ( const TKEY& rtKey, const TVALUE& rtValue );
24
25
TVALUE* Find ( const TKEY& rtKey ) const;
26
27
bool Remove ( const TKEY& rtKey );
28
void RemoveAll ();
29
30
TVALUE* GetFirst ( TKEY* ptKey ) const;
31
TVALUE* GetNext ( TKEY* ptKey ) const;
32
33
int (*UserHashFunction) ( const TKEY& );
34
35
private:
36
class HashItem
37
{
38
public:
39
TKEY m_tKey;
40
TVALUE m_tValue;
41
HashItem* m_pkNext;
42
};
43
44
int HashFunction ( const TKEY& rtKey ) const;
45
46
int m_iTableSize;
47
int m_iQuantity;
48
HashItem** m_apkTable;
49
50
mutable int m_iIndex;
51
mutable HashItem* m_pkItem;
52
};
53
54
#include "THashTable.inl"
55
}
56
#endif//_HASHTABLE_HPP_
THashTable.inl
1
template <class TKEY, class TVALUE>
2
THashTable <TKEY, TVALUE> ::THashTable( int iTableSize )
3

{
4
assert( iTableSize > 0);
5
6
m_iTableSize = iTableSize;
7
m_iQuantity = 0;
8
m_iIndex = 0;
9
m_pkItem = 0;
10
m_apkTable = NEW HashItem*[m_iTableSize];
11
12
memset(m_apkTable, 0, m_iTableSize * sizeof(HashItem*));
13
UserHashFunction = 0;
14
}
15
//-------------------------------------------------------------------------------
16
template <class TKEY, class TVALUE>
17
THashTable<TKEY, TVALUE> ::~THashTable()
18

{
19
RemoveAll();
20
DELETE [] m_apkTable;
21
}
22
//-------------------------------------------------------------------------------
23
template <class TKEY, class TVALUE>
24
int THashTable<TKEY, TVALUE> ::GetQuantity () const
25

{
26
return m_iQuantity;
27
}
28
//-------------------------------------------------------------------------------
29
template <class TKEY, class TVALUE>
30
bool THashTable<TKEY, TVALUE> ::Insert( const TKEY& rtKey,
31
const TVALUE& rtValue )
32

{
33
int iIndex = HashFunction( rtKey );
34
HashItem* pkItem = m_apkTable[iIndex];
35
36
while (pkItem)
37
{
38
if (rtKey == pkItem->m_tKey)
39
{
40
return false;
41
}
42
43
pkItem = pkItem->m_pkNext;
44
}
45
46
pkItem = NEW HashItem;
47
pkItem->m_tKey = rtKey;
48
pkItem->m_tValue = rtValue;
49
pkItem->m_pkNext = m_apkTable[iIndex];
50
m_apkTable[iIndex] = pkItem;
51
++m_iQuantity;
52
53
return true;
54
}
55
//-------------------------------------------------------------------------------
56
template <class TKEY, class TVALUE>
57
TVALUE* THashTable<TKEY, TVALUE> ::Find( const TKEY& rtKey ) const
58

{
59
60
int iIndex = HashFunction( rtKey );
61
HashItem* pkItem = m_apkTable[iIndex];
62
63
while( pkItem )
64
{
65
if (rtKey == pkItem->m_tKey )
66
{
67
return &pkItem->m_tValue;
68
}
69
70
pkItem = pkItem->m_pkNext;
71
}
72
return 0;
73
}
74
//-------------------------------------------------------------------------------
75
template <class TKEY, class TVALUE>
76
bool THashTable<TKEY, TVALUE> ::Remove ( const TKEY& rtKey)
77

{
78
int iIndex = HashFunction( rtKey );
79
HashItem* pkItem = m_apkTable[iIndex];
80
81
if (!pkItem)
82
{
83
return false;
84
}
85
86
if (rtKey == pkItem->m_tKey)
87
{
88
HashItem* pkSave = pkItem;
89
m_apkTable[iIndex] = pkItem->m_pkNext;
90
DELETE pkSave;
91
--m_iQuantity;
92
93
return true;
94
}
95
96
HashItem* pkPrev = pkItem;
97
HashItem* pkCurr = pkItem->m_pkNext;
98
while ( pkPrev && rtKey != pkCurr->m_tKey)
99
{
100
pkPrev = pkCurr;
101
pkCurr = pkCurr->m_pkNext;
102
}
103
104
if (pkCurr)
105
{
106
pkPrev->m_pkNext = pkCurr->m_pkNext;
107
DELETE pkCurr;
108
m_iQuantity--;
109
return true;
110
}
111
112
return false;
113
}
114
//-------------------------------------------------------------------------------
115
template <class TKEY, class TVALUE>
116
void THashTable<TKEY,TVALUE> ::RemoveAll ()
117

{
118
if (m_iQuantity > 0)
119
{
120
for (int iIndex = 0; iIndex < m_iTableSize; iIndex++)
121
{
122
while (m_apkTable[iIndex])
123
{
124
HashItem* pkSave = m_apkTable[iIndex];
125
m_apkTable[iIndex] = m_apkTable[iIndex]->m_pkNext;
126
DELETE pkSave;
127
if (--m_iQuantity == 0)
128
{
129
return;
130
}
131
}
132
}
133
}
134
}
135
//-------------------------------------------------------------------------------
136
template <class TKEY, class TVALUE>
137
TVALUE* THashTable<TKEY,TVALUE> ::GetFirst ( TKEY* ptKey ) const
138

{
139
if (m_iQuantity > 0)
140
{
141
for (m_iIndex = 0; m_iIndex < m_iTableSize; m_iIndex++)
142
{
143
if (m_apkTable[m_iIndex])
144
{
145
m_pkItem = m_apkTable[m_iIndex];
146
*ptKey = m_pkItem->m_tKey;
147
return &m_pkItem->m_tValue;
148
}
149
}
150
}
151
152
return 0;
153
}
154
//-------------------------------------------------------------------------------
155
template <class TKEY, class TVALUE>
156
TVALUE* THashTable<TKEY,TVALUE> ::GetNext ( TKEY* ptKey ) const
157

{
158
if (m_iQuantity > 0)
159
{
160
m_pkItem = m_pkItem->m_pkNext;
161
if (m_pkItem)
162
{
163
*ptKey = m_pkItem->m_tKey;
164
return &m_pkItem->m_tValue;
165
}
166
167
for (m_iIndex++; m_iIndex < m_iTableSize; m_iIndex++)
168
{
169
if (m_apkTable[m_iIndex])
170
{
171
m_pkItem = m_apkTable[m_iIndex];
172
*ptKey = m_pkItem->m_tKey;
173
return &m_pkItem->m_tValue;
174
}
175
}
176
}
177
178
return 0;
179
}
180
//-------------------------------------------------------------------------------
181
template <class TKEY, class TVALUE>
182
int THashTable<TKEY,TVALUE> ::HashFunction (const TKEY& rtKey) const
183

{
184
if (UserHashFunction)
185
{
186
return (*UserHashFunction)(rtKey);
187
}
188
189
// 默認Hash 函數 采用黃金分割
190
static double s_dHashMultiplier = 0.5*(sqrt(5.0)-1.0);
191
unsigned int uiKey;
192
System::Memcpy(&uiKey, sizeof(unsigned int), &rtKey, sizeof(unsigned int));
193
uiKey %= m_iTableSize;
194
double dFraction = fmod(s_dHashMultiplier*uiKey,1.0);
195
return (int)floor(m_iTableSize*dFraction);
196
}
197
//-------------------------------------------------------------------------------
198
199
// end