2012年9月2日
1.軟件p4, vs, vim, cscope.
fix step:
先reproduce
再確定出現問題的函數,行號
分析原因
fix
vs中遠程調試,指定ip,port, 同時在遠程主機上要同意遠程調試,接受指定user。要保證code與exe文件一致。
設定斷點,觀察debug輸入,與預想的有什么不同。觀察bt,thread stack 找到出現問題的函數或者行號。
p4 中的check out, commit, diff, opened, edit, sync命令和作用。
submit step:merge changlist (from branch) (to branch)
check diff
resolve
make (so make sure the code is right)
submit (add comments)
set the bug state in Web
vim:熟悉了各種命令。
cscope,ctag:在linux中瀏覽代碼很有用,可以找到function definition, struct definition, calling function, called function.
在一個目錄中建立cscope database, 可以再主目錄建立,之后只在這里使用cscope,就能找到全部引用。
linux, lib 文件的使用。 有.a 和.o的lib文件。
2.code style.
文件命名要有層次感。例如,snape_webserver_msg.c, snape_webserver_thread.c, snape_webserver_log.c, snape_client_msg.c,,,
函數命名也要有層次感, 例如,snape_webserver_msg_set_connection(), snape_webserver_thread_create(),snape_client_request_get_pic().
變量命名也要有層次感和意義。
出錯處理的專門函數。
debug level:debug,info, basic, webserver, client,,,
3.函數的定義與瀏覽。
call graph definition.
函數名字最好能夠顯示出函數調用的graph。
使用hash table 保存大量類型的數據。
使用內容的md5作為這個內容的id,就可以完美配合hash table。
4.thread, process
windows 中, object , event, cs
cs同步速度較快,但是使用cs容易進入deadlock狀態。因為在等待進入cs時,無法設定超時值。
互斥對象與內核對象屬于內核對象,利用內核對象進行線程同步,同步速度較慢,但這種方式可在多個進程的各個對象之間進行同步。
event分為人工重置與自動重置事件,兩者在細節上,不同。
5.cache implementation
client端可以使用類似os中,cache 與 虛擬內存的方法。b_modified表示是否經過更改,從而是否需要更新。每次只去拿新的東西。
而在server端,可以使用內容的MD5判斷是否需要處理來到的內容,可以用多層次的MD5來更加去improve performance。例如用總體的md5,和各個部分的md5.
先判斷總體MD5,如果改變,在去檢查部分md5.如果沒有改變,就整個都可以丟棄,不去處理。
design is very important. 代碼的執行過程要完全依賴design。
6.select function
異步select, 可以讓線程不去busy waiting。
但是如果需要傳輸內容,需要trigger signal,讓它不用等到timeout,就能發送數據。
7.timestamp/timeout/ ts_diff
可以用ts_diff去同步時間。
使用timeout來防止任意操作的無限制等待
使用timestamp來決定哪一個更新。不要用counter。(無法同步,無法控制單一性)
8.xml api
在傳送數據方面,可以使用xml
9.performace/memory usage
mm tools.
2012年8月17日
Suppose I have the following C code.
unsigned int u = 1234;
int i = -5678;
unsigned int result = u + i;
What implicit conversions are going on here, and is this code safe for all values of u
and i
? (Safe, in the sense that even though result in this example will overflow to some huge positive number, I could cast it back to an int and get the real result.)
Answer:
Short Answer
Your i
will be converted to an unsigned integer by adding UINT_MAX + 1
, then the addition will be carried out with the unsigned values, resulting in a large result
(depending on the values of u
andi
).
Long Answer
According to the C99 Standard:
6.3.1.8 Usual arithmetic conversions
- If both operands have the same type, then no further conversion is needed.
- Otherwise, if both operands have signed integer types or both have unsigned integer types, the operand with the type of lesser integer conversion rank is converted to the type of the operand with greater rank.
- Otherwise, if the operand that has unsigned integer type has rank greater or equal to the rank of the type of the other operand, then the operand with signed integer type is converted to the type of the operand with unsigned integer type.
- Otherwise, if the type of the operand with signed integer type can represent all of the values of the type of the operand with unsigned integer type, then the operand with unsigned integer type is converted to the type of the operand with signed integer type.
- Otherwise, both operands are converted to the unsigned integer type corresponding to the type of the operand with signed integer type.
In your case, we have one unsigned int (u
) and signed int (i
). Referring to (3) above, since both operands have the same rank, your i
will need to be converted to an unsigned integer.
6.3.1.3 Signed and unsigned integers
- When a value with integer type is converted to another integer type other than _Bool, if the value can be represented by the new type, it is unchanged.
- Otherwise, if the new type is unsigned, the value is converted by repeatedly adding or subtracting one more than the maximum value that can be represented in the new type until the value is in the range of the new type.
- Otherwise, the new type is signed and the value cannot be represented in it; either the result is implementation-defined or an implementation-defined signal is raised.
Now we need to refer to (2) above. Your i
will be converted to an unsigned value by adding UINT_MAX + 1
. So the result will depend on how UINT_MAX
is defined on your implementation. It will be large, but it will not overflow, because:
6.2.5 (9)
A computation involving unsigned operands can never overflow, because a result that cannot be represented by the resulting unsigned integer type is reduced modulo the number that is one greater than the largest value that can be represented by the resulting type.
Bonus: Arithmetic Conversion Semi-WTF
#include <stdio.h>
int main(void)
{
unsigned int plus_one = 1;
int minus_one = -1;
if(plus_one < minus_one)
printf("1 < -1");
else
printf("boring");
return 0;
}
You can use this link to try this online: http://codepad.org/yPhYCMFO
Bonus: Arithmetic Conversion Side Effect
Arithmetic conversion rules can be used to get the value of UINT_MAX
by initializing an unsigned value to -1
, ie:
unsigned int umax = -1; // umax set to UINT_MAX
This is guaranteed to be portable regardless of the signed number representation of the system because of the conversion rules described above. See this SO question for more information: Is it safe to use -1 to set all bits to true?
2012年6月25日
1.標示符名稱的限制
ANSI C標準只保證了C實現必須能夠
區別出前6個字符不同的外部名稱。而且這個定義中并
沒有區分大寫字母與其對應的小寫字母。因此,編寫可移植程序必須小心這一點。
2.字符是有符號的整數,還是無符號的整數
只有把一個字符值轉換
為一個較大的整數時,才重要。在其他情況下,結果都是:多余的位被簡單的“丟棄”。
在轉換過程中:應該將字符作為有符號數還是無符號數?
如果有符號,編譯器將char數據,擴展到int時候,應該復制符號位。
如果無符號,編譯器只需在多余的位上填充0.
#include <stdio.h>
int main()
{
char c='a';
c=c+40;
// printf("%c\n", -1);
printf("c %d\n", c);
printf("unsigned c %u\n", (unsigned char)c);
}
結果:
c -119
unsigned c 137
說明在gcc中,將char當做有符號數。在c+40的時候,超過了-128~127范圍,因此溢出。如果是無符號char,范圍是0~255.應該是輸出137.
如果編程者關注一個最高位是1的字符是正還是負,可以設置
為無符號字符數。這樣所有編譯器都會轉換為整數時候,填充為0.
3.一個常見錯誤是:如果c是一個字符變量,使用(unsigned)c可以得到與c等價的無符號整數。
這是會失敗的。因為在將c轉換為無符號整數時候,c將首先首先被轉換為int型整數。而此時可能得到非預期的結果。
正確方法是:(unsigned char )c,直接進行轉換。
例如上個例子中,最后一句改為:
printf("unsigned c %u\n", (unsigned )c);
那么結果是:
c -119
unsigned c 4294967177
c被先轉換為int型-119,再求他的無符號表達形式,
4294967177 4.移位運算符
1.向右移位時,空出的位由0填充,還是由符號位的副本填充。
2.如果是無符號數,用0填充。如果是有符號數,既可以用0也可以用符號位的副本。(如果關注右移時候空出的位,可以聲明為無符號類型,那么空出的位都會被設置為0)
如果被移位對象為n位,那么移位計數
必須大于或等于0,而嚴格小于n.即使C實現將符號位復制到空出的位中,有符號數的向右移位,
也并不等于除以2的某次冪。例如(-1)>>1結果為-1,而不是-1/2 == 0
5.隨機數最大值,RAND_MAX在limits中定義。我測試結果等于INT_MAX
6.除法運算的截斷
q=a/b;
r=a%b;
假定b>0.
C語言定義只保證q*b+r=a,以及a>=0 且 b>0時,保證|r|<|b|以及r>=0.(如果a<0, 那么
r也可能小于0)例如:
int main() {
// Start typing your code here
cout<<(-3)/2<<endl;
return 0;
}
結果商為-1,余數也為-1
使用預處理器的兩個主要原因:
1.一次修改變量,出現的所有的值都會修改。
講所有常量定義集中在一起。
2.避免函數調用開銷。
3.宏定義注意點
1.不能忽視定義中的空格
2.最好將宏定義中每個參數都用括號括起來。整個表達式的結果頁用括號括起來。
3.確保調用宏的參數中,不存在有副作用的代碼
4.assert宏。可以在出錯信息中包含文件名和斷言失敗處的行號。很有用。
5.宏并不是類型定義。
#define T1 struct foo *
typedef struct foo * T2;
T1 a,b; //struct foo * a, b;
T2 a,b; //a ,b都是指向結構的指針。
1.getchar
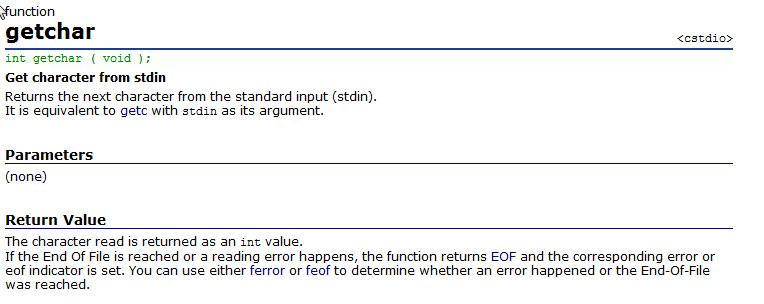
getchar返回整形
#include <stdio.h>
int main()
{
char c;
while( (c=getchar())!=EOF )
putchar(c);
}
應該將c聲明為int。否則,c可能無法容下EOF
2.更新文件
讀操作之后,文件指針會偏移一段。這時候,講文件更新后,寫入源文件之前,應該fseek講文件指針調回去。
3.使用setbuf設置輸出的緩沖區大小。可以是stdout和file
4.正確使用errno檢測錯誤
errno=0;
/*調用庫函數*/
if(返回的錯誤值) //這個錯誤值可能不是由當前這個函數引起的。而是由當前函數,又調用的另外一個函數引起的。
檢查errno;
5.signal處理函數唯一安全,可移植的操作就是打印一條錯誤信息,然后使用longjmp或者exit立即退出程序。
1.如果一個函數僅僅被同一個源文件中的其他函數調用,我們就應該聲明該函數為static
2.extern int n;
在兩外一個文件中: long n;
這是一個無效的程序,因為同一個外部變量名在兩個不同的文件中被聲明為不同的類型。然后大多數c語言實現不能檢測出這種錯誤。
3.一個程序由多個模塊組成,每個模塊都需要知道一個特定的文件名。我們希望能夠做到只在一處改動這個文件名,所有模塊中的文件名就能同時得到更新。
可以,先創建一個文件,叫做file.h,它包含了聲明
extern char filename[];需要用到外部對象filename的每個c源文件都應該加上: #include "file.h";
最后選擇一個C源文件,在其中給出filename的初始值。如在file.c中
#include "file.h";
char filename[]="/etc/passwd";
這樣就保證了filename的類型是正確的。解決了2中的問題。
整數溢出
c語言中存在兩類整數算術運算,有符號運算和無符號運算。在無符號運算里,沒有了符號位,所以是沒有溢出的概念的。
所有的無符號運算都是以2的n次方為模。如果算術運算符的一個操作數是有符號書,另一個是無符號數,那么有符號數
會被轉換為無符號數(表示范圍小的總是被轉換為表示范圍大的),那么溢出也不會發生。但是,當兩個操作數都是有符號數
時,溢出就有可能發生。而且溢出的結果是未定義的。當一個運算的結果發生溢出時,任何假設都是不安全的。
例如,假定a和b是兩個非負的整型變量(有符號),我們需要檢查a+b是否溢出,一種想當然的方式是:
if (a + b < 0)
溢出;
實際上,在現實世界里,這并不能正常運行。當a+b確實發生溢出時,所有關于結果如何的假設均不可靠。比如,在某些
機器的cpu,加法運算將設置一個內部寄存器為四種狀態:正,負,零和溢出。在這種機器上,c編譯器完全有理由實現以上
的例子,使得a+b返回的不是負,而是這個內存寄存器的溢出狀態。顯然,if的判斷會失敗。
一種正確的方式是將a和b都強制轉換為無符號整數:
if ( (unsigned)a + (unsigned)b > INT_MAX)
溢出;
這里的int_max值為有符號整型的最大值。在一般的編譯器里是一個預定義的常量。ANSI C在limits里定義了INT_MAX,值為
2的31次方-1.
不需要用到無符號算數運算的另一種可行方法是:
if (a > INT_MAX - b )
溢出;
2012年6月24日
1.int a[10]; 除了a被用作運算符
sizeof()的參數這一情況,在其他所有的情形中,數組名a都代表指向數組
a中下標為0的元素的指針。因此,int *p=a; //right
int *p=&a; //error, (&a已經是一個指向整個數組的指針)
2.為main函數提供返回值
main()
{}
隱含著main返回整數,
一個返回整數的函數如果返回失敗,實際上隱含返回某個“垃圾”整數,只要該值不被用到,就無關緊要。
然而,在某些情況下,main的返回值卻并非無關緊要,大多數C語言實現通過main的返回值,
來告知操作系統該函數的執行是成功還是失敗。如果一個程序的main函數并不返回任何值,那么
有可能看上去執行失敗。所以最好提供返回值
3.邊界計算與不對稱邊界。
適合c中以下標為0開始的計算。
2012年6月23日
1.y=x/*p; //p指向除數
error.
/*會被當做注釋的開始。應該y=x / *p;
或者y = x/(*p);
2.如果一個整形常量第一個字符為數字0.那么這個常量會被當做8進制數。